Cracking the Code: Exploring Reverse Engineering and MobSF for Mobile App Security
9 min read
June 23, 2024
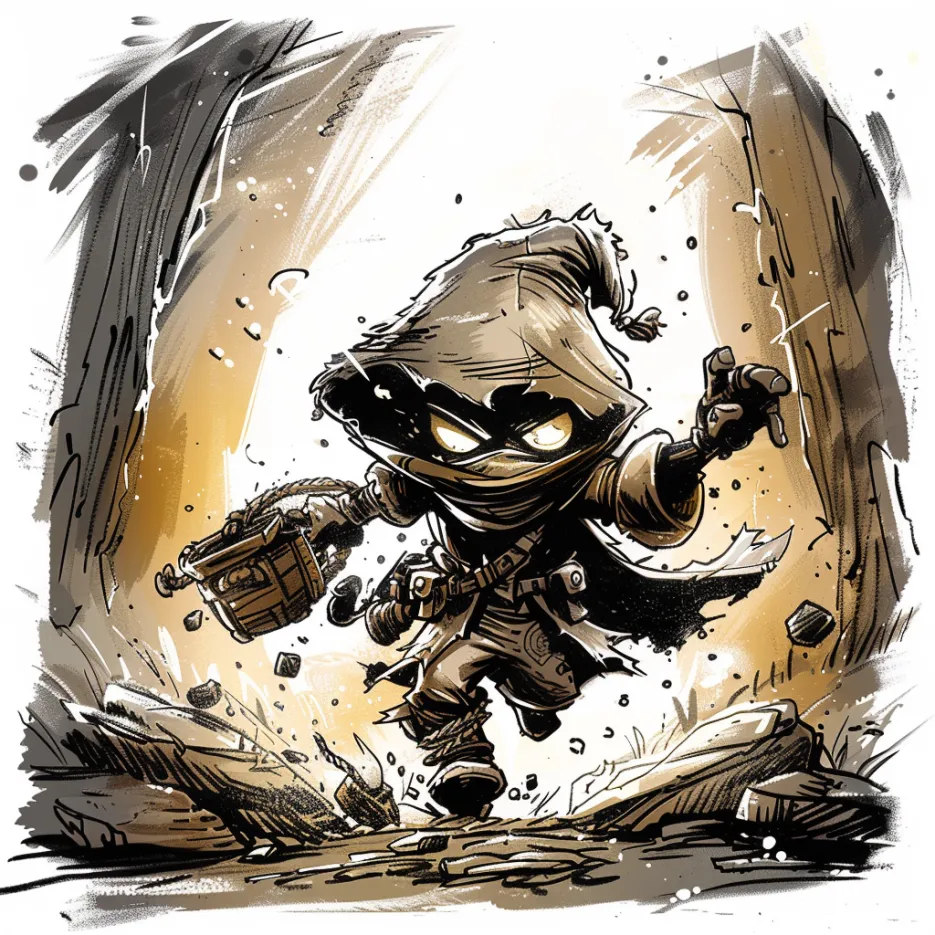
Table of contents
Introduction
In our previous discussions, we delved into the theoretical aspect of creating new JWTs after compromising its secret. Despite this, we faced a challenge where the server's responses were encoded, and the front end of the application displayed no information, leaving us in the dark about the underlying issues. In this chapter, we aim to decode the server's responses by examining the application's code. Additionally, we will introduce MobSF (Mobile Security Framework) as a robust tool for performing automated security analysis of mobile applications. This comprehensive approach will help us gain deeper insights into the application's behavior and enhance its security posture.
Key takeaways from this chapter include:
- Decoding Server Responses: Understanding how to decode the encoded server responses to uncover vital information.
- Reverse Engineering APKs: Techniques to extract and analyze the Java code from APK files.
- Understanding Obfuscation: Insights into obfuscation techniques used in encoding and decoding data.
- Using Frida for Dynamic Analysis: Leveraging Frida scripts to intercept and analyze data in real-time.
- Utilizing MobSF: Introducing MobSF as a powerful tool for static and dynamic analysis of mobile applications.
Reverse Engineering
The first thing we need to do to study the application's code is to obtain the Java code from the APK. To achieve this, we can use apkx, just as we did in the first chapter of this series.
apkx dvba-no-gpu.apk
After obtaining the code, we need to search for the Profile
class to try to understand what is happening.
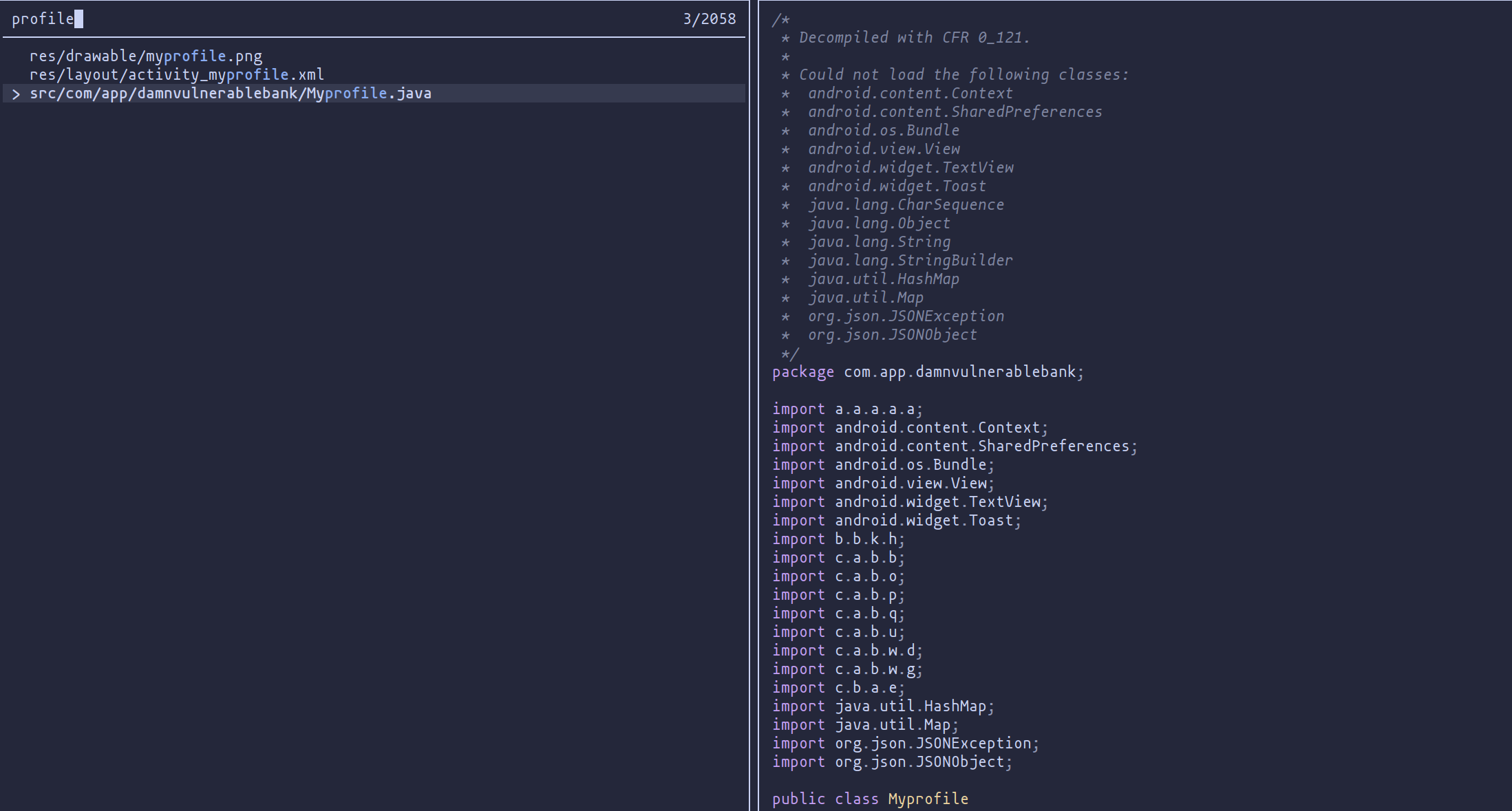
Once we have found the class, we can perform a bit of reverse engineering to discover that the class containing the code to encode the data is e
.
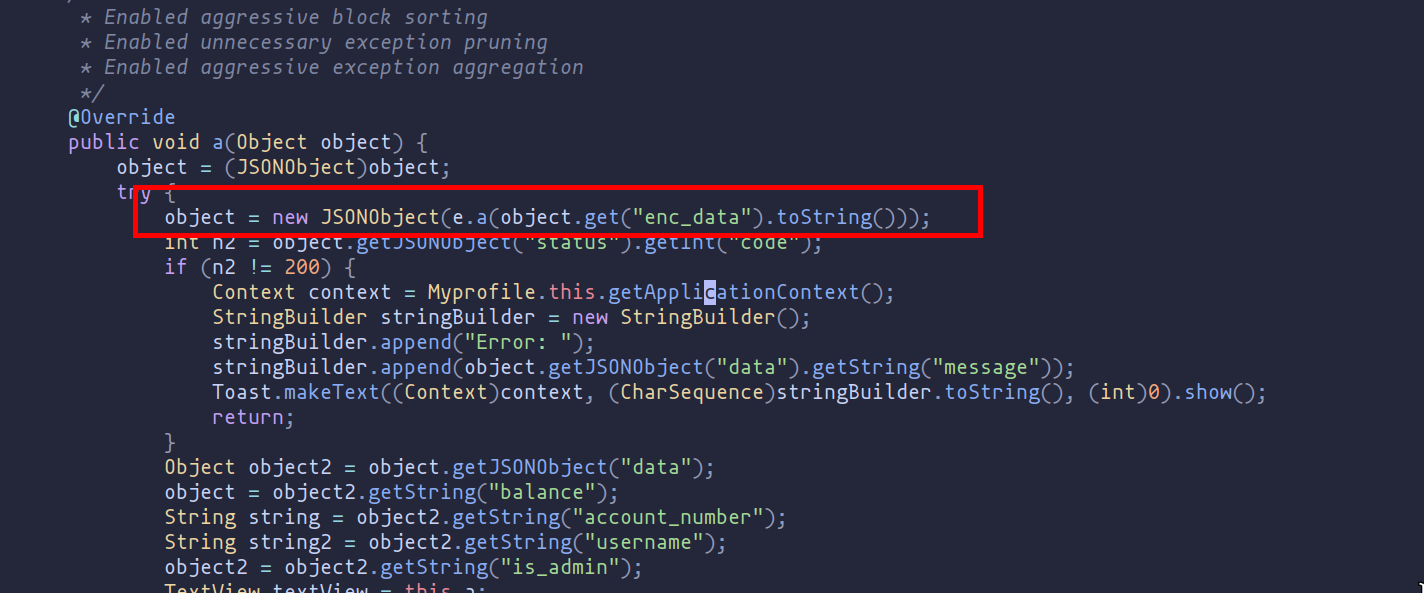
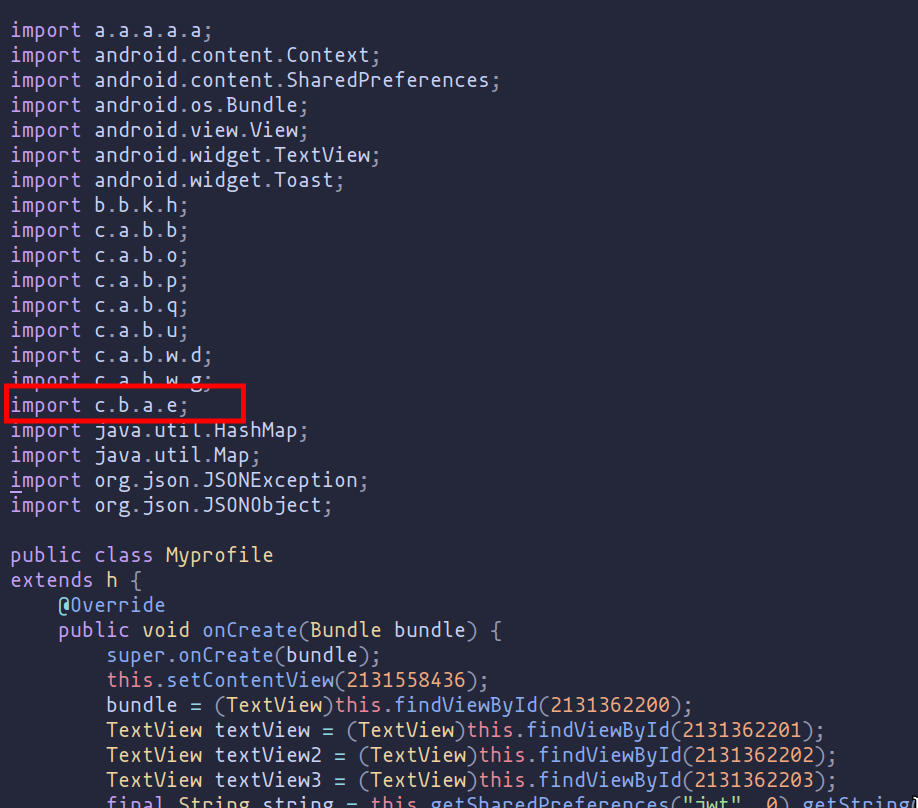
The class e
provides functionality to encode and decode strings using Base64, with an added layer of obfuscation. The obfuscation is achieved by XOR-ing each character of the input string with a repeating sequence of characters from the word "amazing"
.
a
method: Decodes a Base64 encoded string and then obfuscates it.b
method: Obfuscates a string and then encodes it in Base64.c
method: Obfuscates a string using a simple XOR-based technique.
We can deduce that the encoded data is built by first using Base64 encoding and then applying an XOR operation with the key "amazing"
.
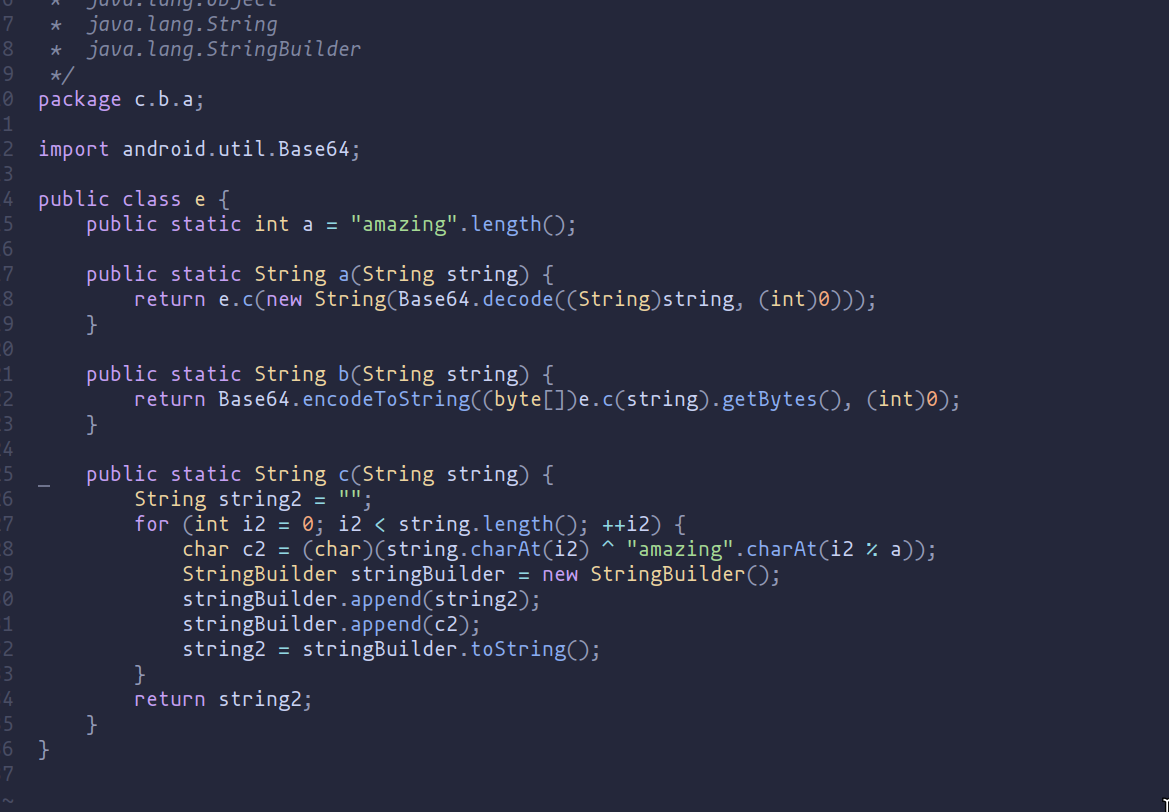
e
With this information, we can develop a Frida script that prints both the encoded data and the decoded data:
// Delay execution to ensure proper Java environment setup
setTimeout(function() {
Java.perform(function() {
var CryptClass = Java.use("c.b.a.e"); // Locate the class c.b.a.e
// Hooking method 'a' to intercept encrypted data
CryptClass.a.implementation = function(encryptedData) {
console.log("Encrypted Data: " + encryptedData); // Log encrypted data
var responseData = this.a(encryptedData); // Execute original method
console.log("Response Data: " + responseData); // Log decrypted response data
return responseData; // Return the original method's result
};
});
}, 10); // Wait 10ms before executing
// Delay execution to ensure proper Java environment setup
setTimeout(function() {
Java.perform(function() {
var CryptClass = Java.use("c.b.a.e"); // Locate the class c.b.a.e
// Hooking method 'b' to intercept request data
CryptClass.b.implementation = function(requestData) {
console.log("Request Data: " + requestData); // Log plaintext request data
var encryptedResponse = this.b(requestData); // Execute original method
console.log("Encrypted Data: " + encryptedResponse); // Log encrypted response data
return encryptedResponse; // Return the original method's result
};
});
}, 10); // Wait 10ms before executing
As you can see in the following image, now, when we enter the profile section of the application, Frida will report both the encoded and decoded data.
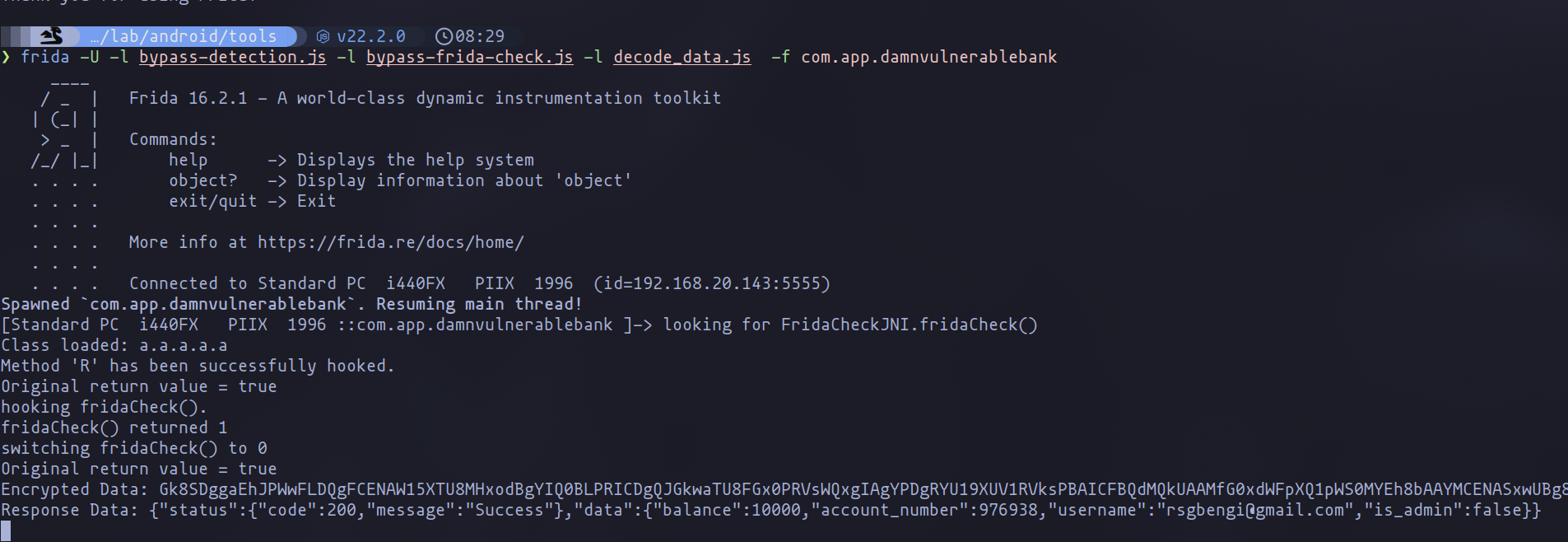
However, for some reason, when I change the user's JWT, the data does not appear in the terminal using Frida. Therefore, I decided to develop a small Python script to decode the data. This way, I can get the encoded data from a response after changing the JWT and decode the information.
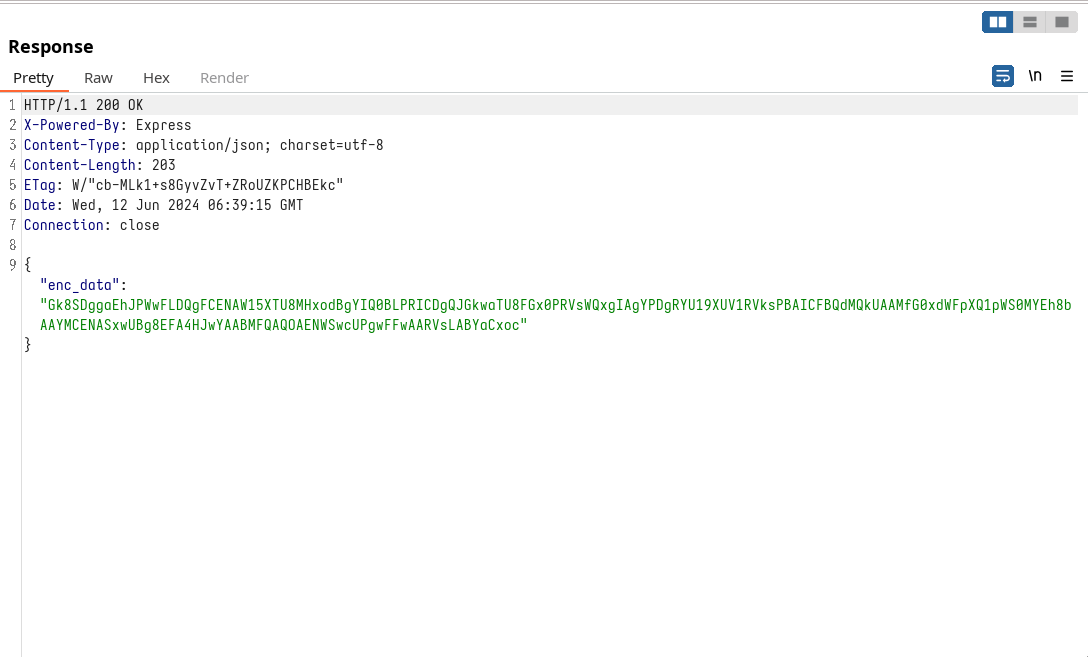
{"enc_data":"Gk8SDggaEhJPWwFLDQgFCENAW15XTU8MHxodBgYIQ0BLPRICDgQJGkwaTU8FGx0PRVsWQxgIAgYPDgRYU19XUV1RVksPBAICFBQdMQkUAAMfG0xdWFpXQ1pWS0MYEh8bAAYMCENASxwUBg8EFA4HJwYAABMFQAQOAENWSwcUPgwFFwAARVsLABYaCxoc"}
import base64
def decode_base64_and_xor(encoded_string):
"""
Decodes a Base64 encoded string and then applies an XOR operation with the string "amazing".
:param encoded_string: The Base64 encoded string.
:return: The decoded string.
"""
# Decode the Base64 string
decoded_bytes = base64.b64decode(encoded_string)
decoded_string = decoded_bytes.decode('utf-8')
# Apply the XOR operation with the string "amazing"
key = "amazing"
result = []
for i in range(len(decoded_string)):
xor_char = chr(ord(decoded_string[i]) ^ ord(key[i % len(key)]))
result.append(xor_char)
return ''.join(result)
# Example usage
if __name__ == "__main__":
encoded_string = "your_encoded_string_here" # Replace this with your encoded string
decoded_string = decode_base64_and_xor(encoded_string)
print("Decoded string:", decoded_string)
As you can see in the following image, in this case, we have successfully decoded the data and discovered the profile information of another user, which in this case is beru
.
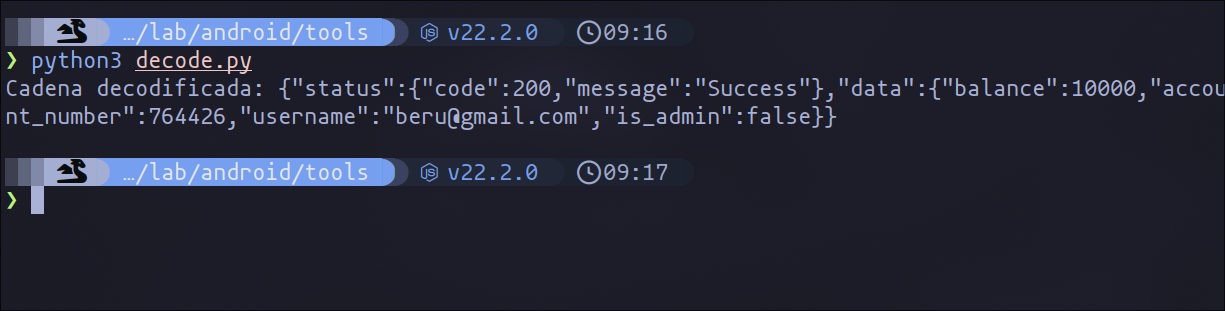
This application has a default admin user, so we can also try to find out their data. By changing the JWT username from "[email protected]"
to "admin"
and setting "is_admin"
to "true"
, we can impersonate the admin user.
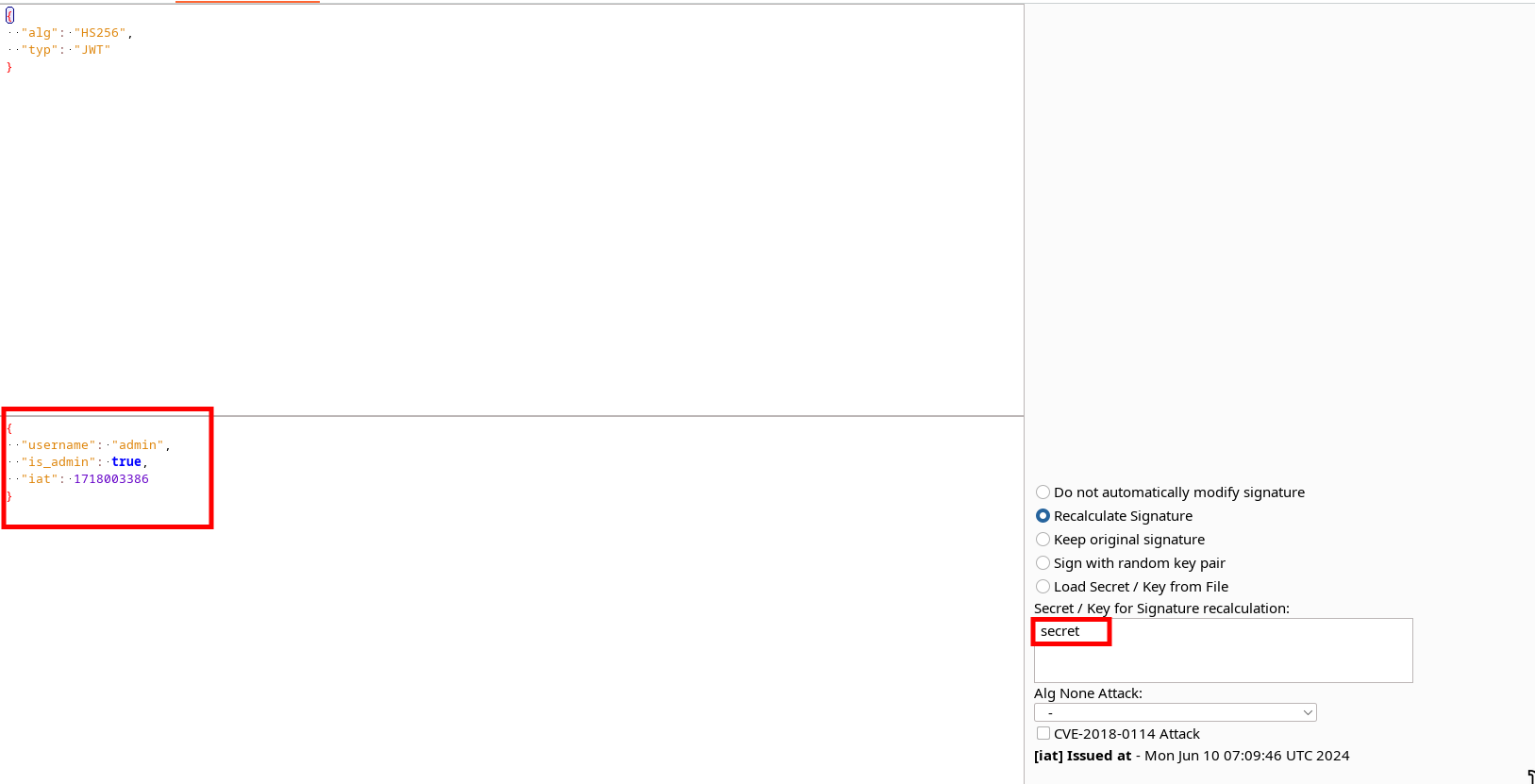

Understanding MobSF: A Powerful Mobile Security Framework
Moving on from the previous section where we discussed reverse engineering, let's now dive into what MobSF is and why it’s an essential tool for mobile security.
What is MobSF?
MobSF (Mobile Security Framework) is an open-source security framework designed to perform automated security analysis of mobile applications. It supports both Android and iOS platforms, making it a versatile tool for mobile app developers and security analysts alike. Whether you have an APK (Android Package) or an IPA (iOS App Store Package), MobSF can dissect it and provide detailed insights into its security posture.
Key Features of MobSF:
- Static Analysis: MobSF performs static analysis by decompiling the application code and inspecting it for vulnerabilities. This includes identifying insecure coding practices, potential data leaks, and other security flaws without executing the app.
- Dynamic Analysis: For a more in-depth assessment, MobSF can also run the app in a controlled environment to observe its behavior in real-time. This dynamic analysis helps in identifying runtime issues, such as network security concerns and improper use of APIs.
- API Analysis: MobSF examines the APIs used by the application to detect any insecure or outdated APIs that might expose the app to security risks.
- Malware Analysis: The framework includes malware analysis capabilities, allowing it to detect malicious code that could compromise the device or the data it handles.
- User-Friendly Reports: After completing its analysis, MobSF generates comprehensive and user-friendly reports. These reports highlight the identified vulnerabilities and provide recommendations for remediation, making it easier for developers to enhance the security of their applications.
Installing MobSF with Docker
The process of installing MobSF (Mobile Security Framework) is quite easy, thanks to Docker. You only need to execute the following code:
# Pulling the image
docker pull opensecurity/mobile-security-framework-mobsf:latest
# Running the docker container
docker run -it --name mobsf -p 8000:8000 opensecurity/mobile-security-framework-mobsf:latest
Steps to Install MobSF
- Pull the MobSF Docker Image: The first step is to pull the latest MobSF Docker image from the Docker repository. This can be done using the
docker pull
command:
docker pull opensecurity/mobile-security-framework-mobsf
- Run the Docker Container: Once the image is downloaded, you can run the MobSF Docker container. The
-it
flag runs the container in interactive mode, and the-p 8000:8000
flag maps port 8000 on your local machine to port 8000 on the Docker container, making the MobSF web interface accessible viahttp://localhost:8000
:
docker run -it -p 8000:8000 opensecurity/mobile-security-framework-mobsf:latest
Starting Your First Scan
Once MobSF is installed and running, initiating your first scan is quite simple. Follow these steps:
- Access the MobSF Web Interface: Open your web browser and go to
http://localhost:8000
. Log in with the credentials:
Username: mobsf
Password: mobsf
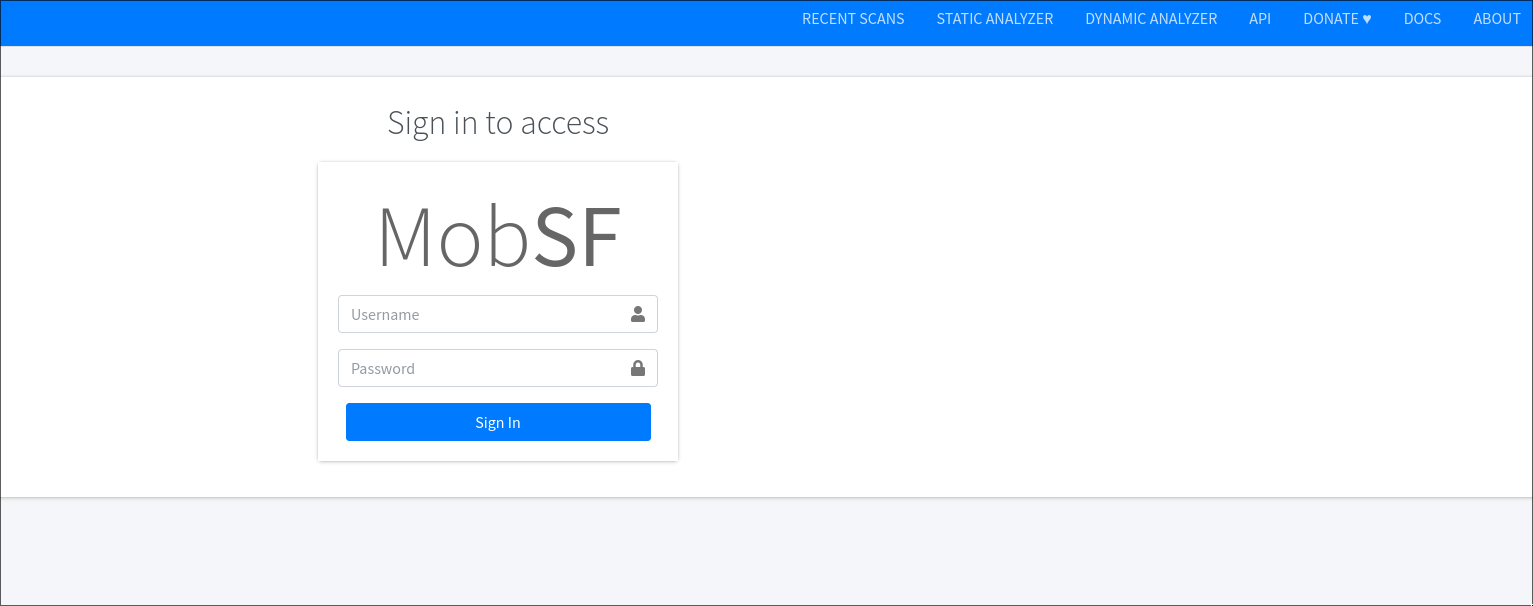
- Select "Static Analyzer": From the MobSF dashboard, navigate to the "Static Analyzer" option. This tool allows you to perform a comprehensive analysis of your APK.

- Upload Your APK: Click on the upload button and select the APK file you want to analyze. MobSF will start the static analysis automatically once the APK is uploaded.
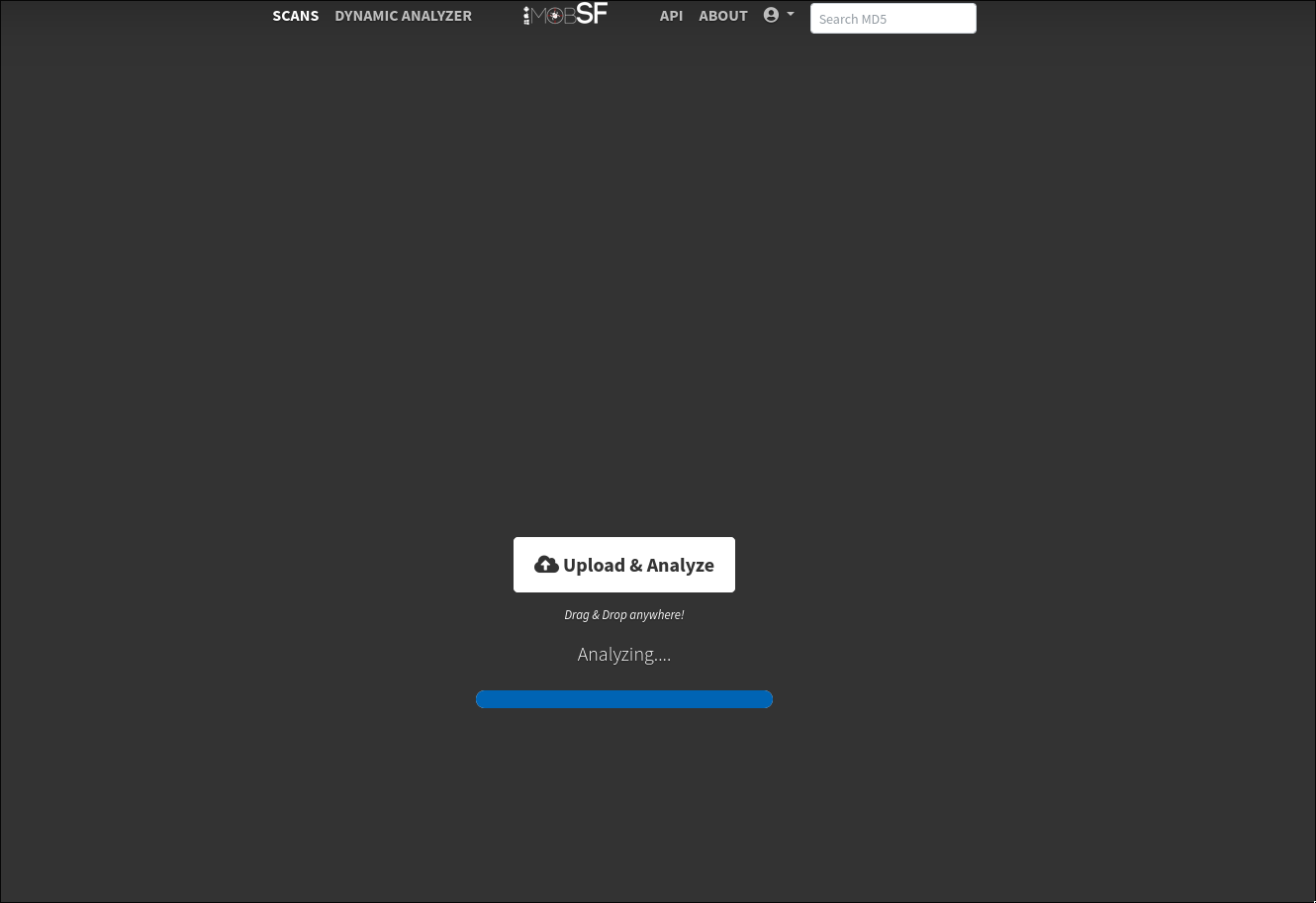
Analyzing Your First Scan
The Static Analyzer will dissect the APK, examining various components such as permissions, code structure, and security configurations. After the analysis is complete, MobSF will provide a detailed report highlighting potential vulnerabilities and security issues.
When the analyzer finishes, you will see a comprehensive report generated by MobSF. It is highly recommended to thoroughly review all sections of the report, as they contain valuable insights into the application's security posture.
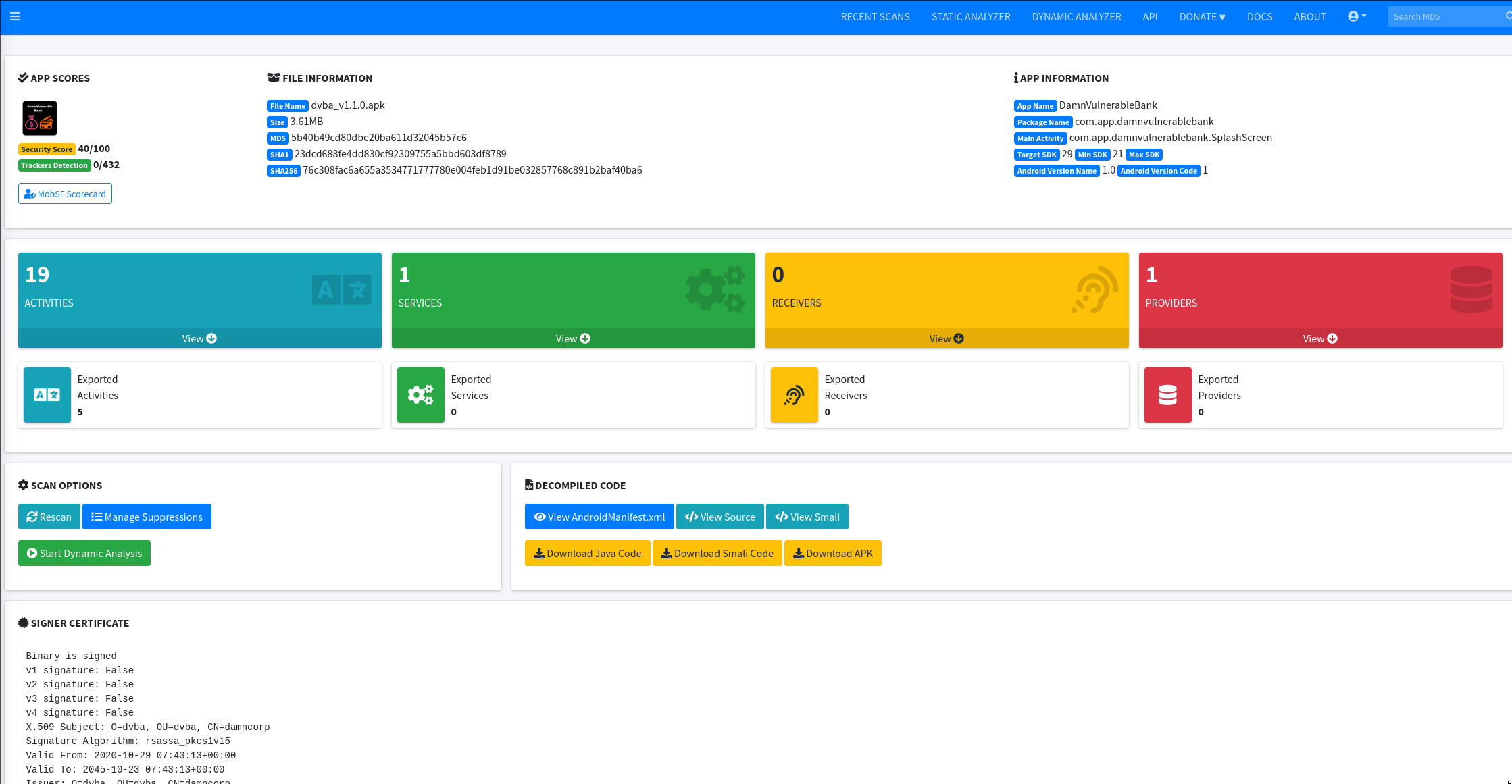
Key Sections of the Report
Security Analysis: The Security Analysis section is particularly crucial as it encompasses a wide range of information focused on both the configuration and code of the application. Some of the most useful tabs within this section include:
- Network Configuration Analysis: This tab reviews the security of the application's network communications. Similar to tools like Testssl for web applications, it assesses HTTPS implementation and other network security practices. Here, you can identify issues such as weak SSL/TLS configurations, insecure transmission of data, and other network-related vulnerabilities.
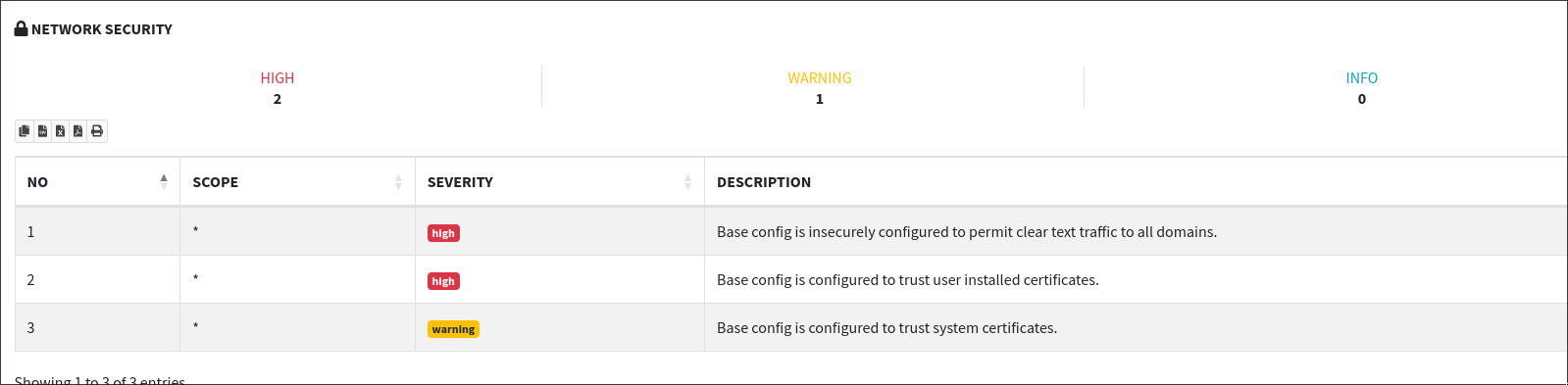
- Manifest Configuration Analysis: The manifest file is essential for setting up the application's permissions and components. This tab analyzes the manifest for potential misconfigurations and over-permissive settings that could lead to security risks. You can download the manifest directly from MobSF for a detailed inspection.
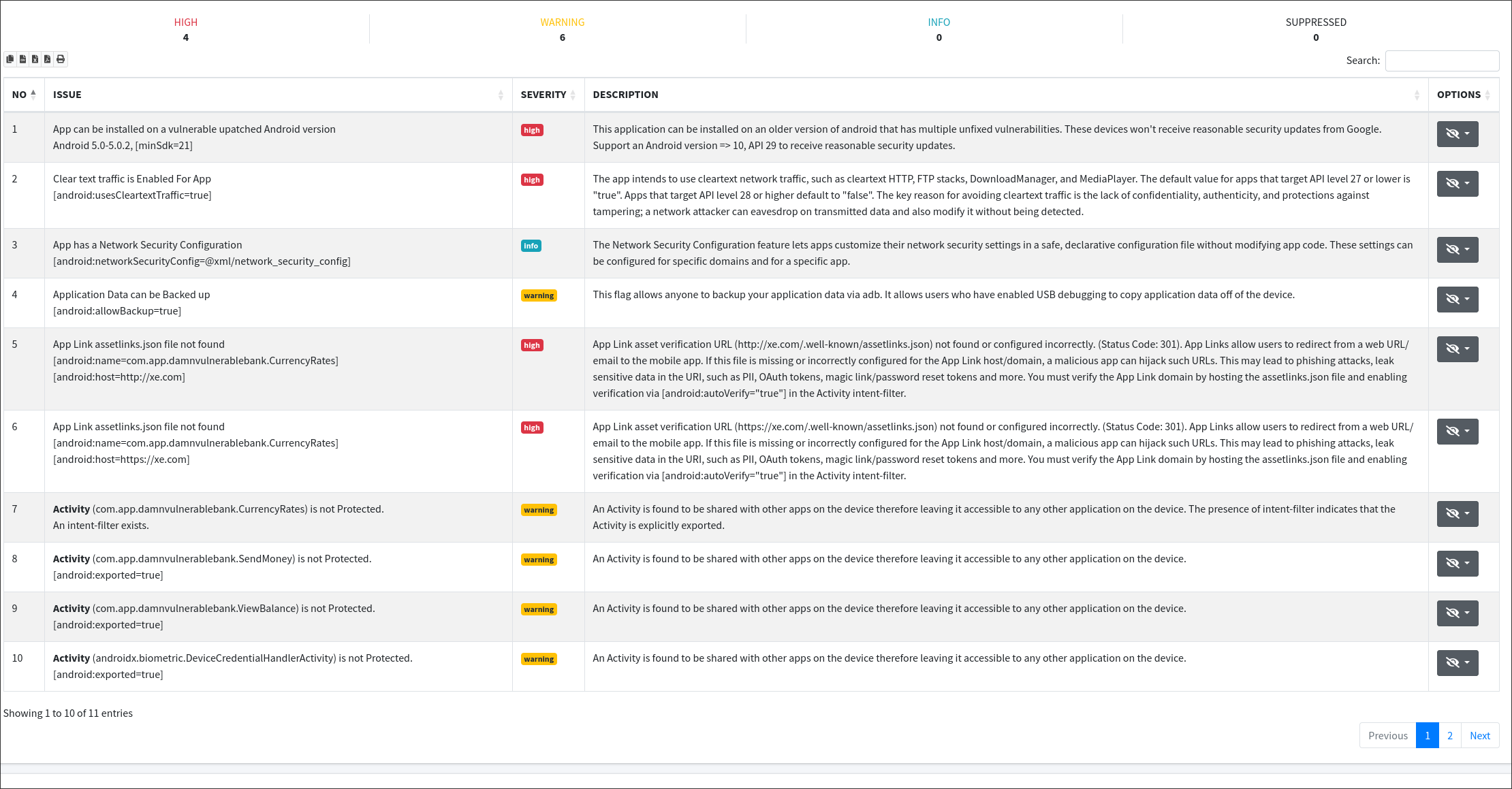
- Code Analysis: This tab delves into the source code, identifying insecure coding practices and potential vulnerabilities such as hardcoded credentials, insecure data handling, and possible SQL injection points. Code analysis often uncovers subtle issues that might not be apparent in other sections.
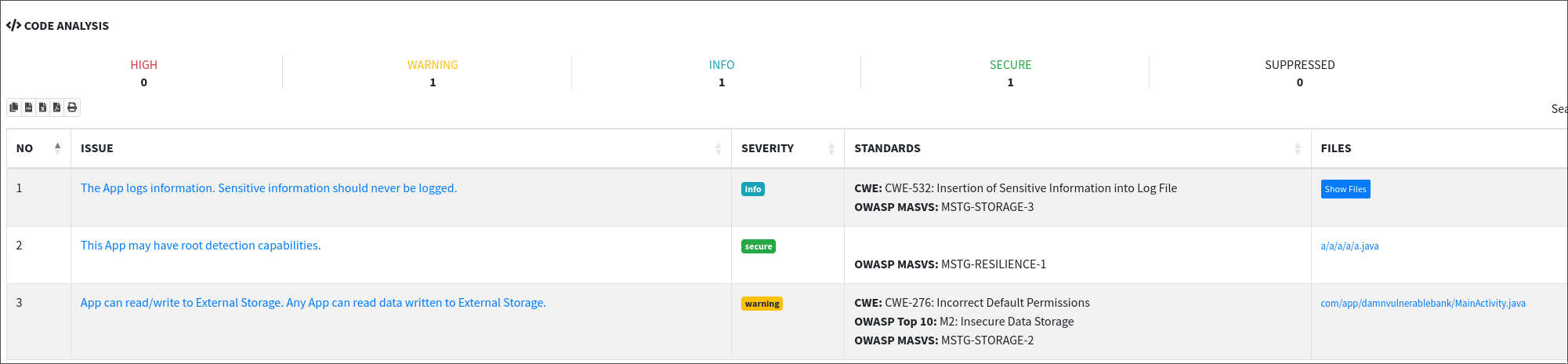
Reconnaissance: The Reconnaissance section is invaluable for identifying exposed sensitive information. Key areas to examine include:
- Hardcoded Secrets: This part of the report identifies any hardcoded secrets within the application, such as API keys, passwords, and tokens. These can be critical security issues as they might provide attackers with direct access to backend systems or services.
- Email Addresses: The report also highlights any email addresses found within the APK. These can sometimes be used for phishing attacks or to gather more information about the application's developers and stakeholders.
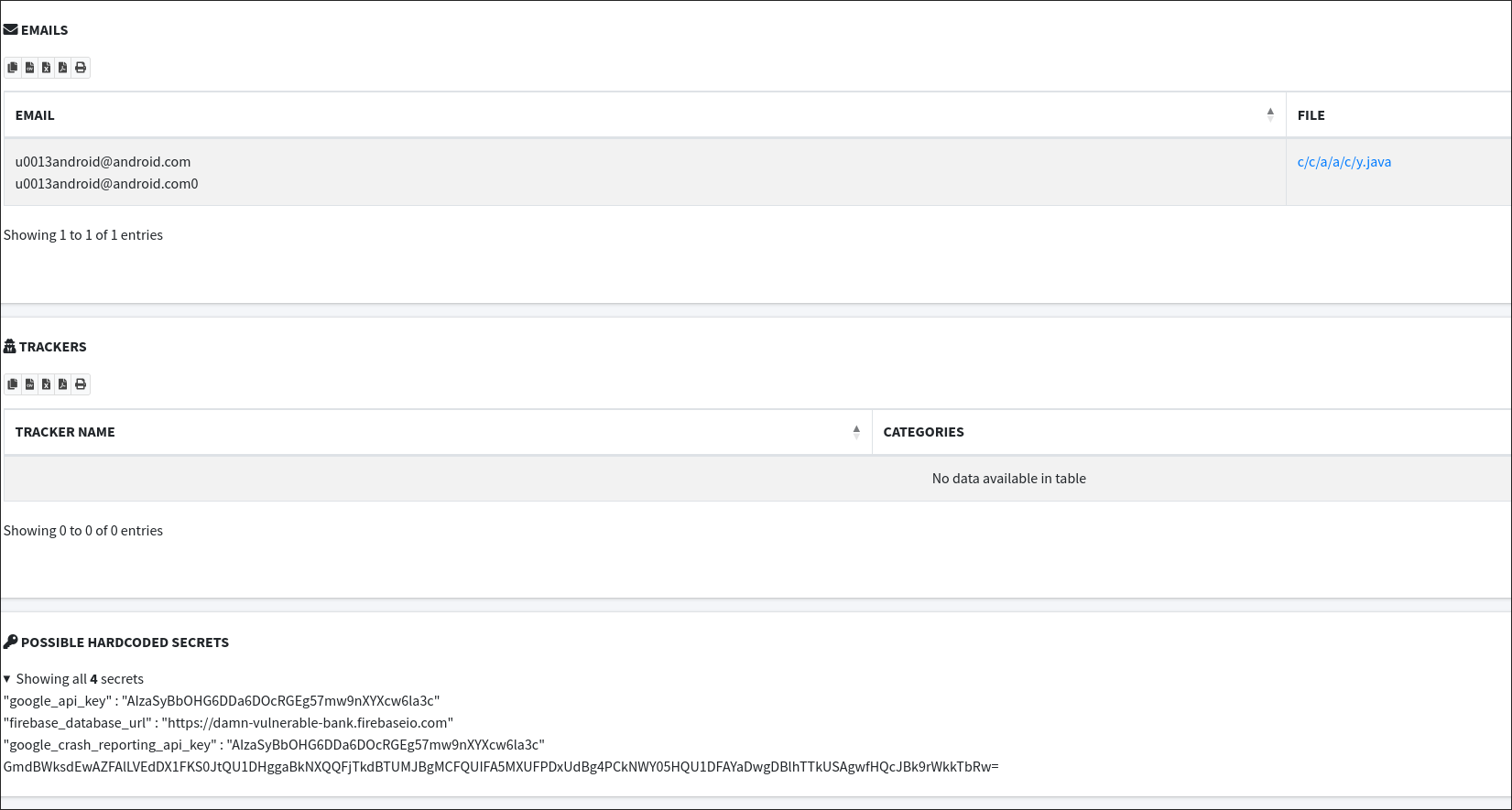
Additional Insights: MobSF provides a plethora of other details that can help enhance your application's security. This includes:
- File Analysis: Inspect all files within the APK package for malicious content, unauthorized modifications, and security risks. Configuration files, assets, and embedded libraries are all analyzed to ensure they do not introduce vulnerabilities.
- Binary Analysis: The compiled binary is scrutinized to uncover any hidden malicious code or unintended functionality. This involves reverse engineering and examining the binary for signs of tampering or embedded malware.
Conclusion
In this chapter, we tackled the challenge of decoding server responses and understanding the application's behavior through reverse engineering. By extracting and analyzing the Java code from the APK, we uncovered how the application encodes and decodes data, providing valuable insights into its inner workings. This process highlighted the importance of reverse engineering in identifying and addressing potential security issues.
Moving forward, we introduced MobSF (Mobile Security Framework) as a powerful tool for performing automated security analysis of mobile applications. MobSF's capabilities in both static and dynamic analysis allowed us to gain a comprehensive understanding of the application's security posture. By utilizing MobSF, we were able to identify vulnerabilities, examine network configurations, and analyze code for insecure practices.
References
- Muellerberndt, B. "APKX: One-Step APK Decompilation With Multiple Backends." GitHub Repository. Available at: https://github.com/muellerberndt/apkx
- Frida: A world-class dynamic instrumentation toolkit. "Frida Documentation." Available at: https://frida.re
- Base64 Encoding and Decoding. "RFC 4648 - The Base16, Base32, and Base64 Data Encodings." Available at: https://tools.ietf.org/html/rfc4648
- XOR Obfuscation. "XOR Cipher - Wikipedia." Available at: https://en.wikipedia.org/wiki/XOR_cipher
- MobSF (Mobile Security Framework). "MobSF Documentation." Available at: https://mobsf.github.io/docs/
- Docker Documentation. "Get Started with Docker." Available at: https://docs.docker.com/get-started/
- JSON Web Tokens. "JWT.io - Introduction to JSON Web Tokens." Available at: https://jwt.io/introduction/
- TestSSL.sh. "TestSSL.sh Documentation." Available at: https://testssl.sh/
Chapters
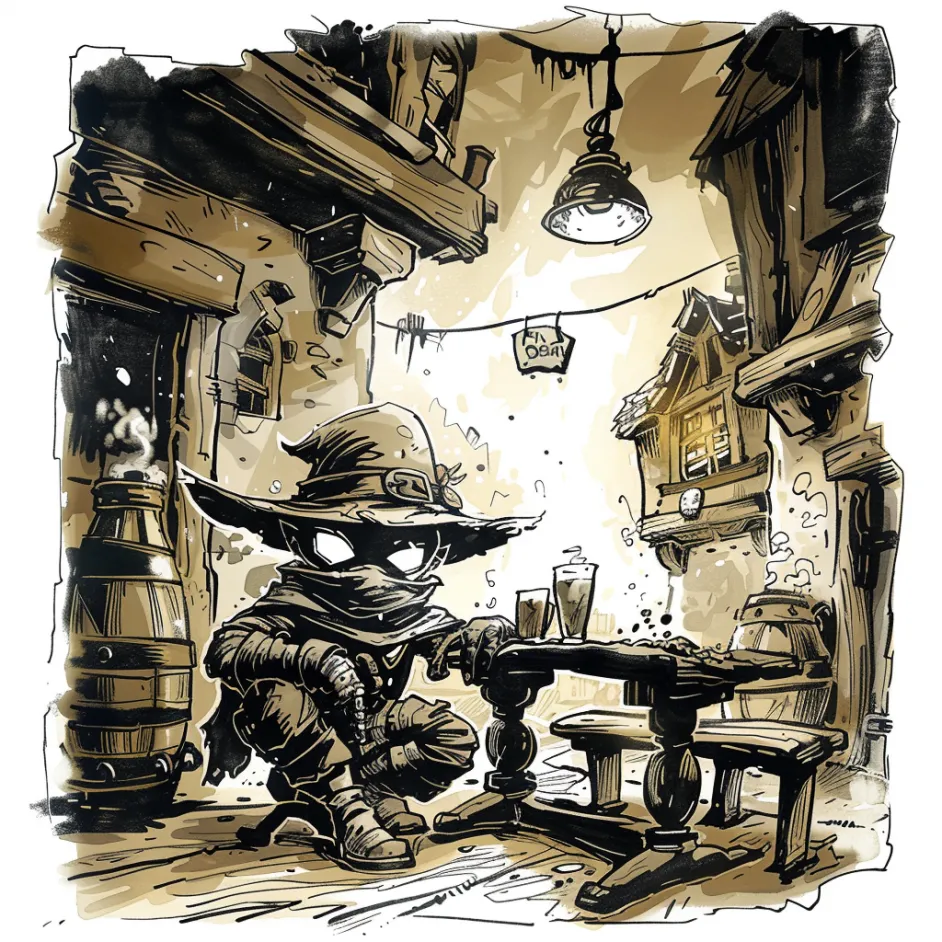
Previous chapter
Next chapter
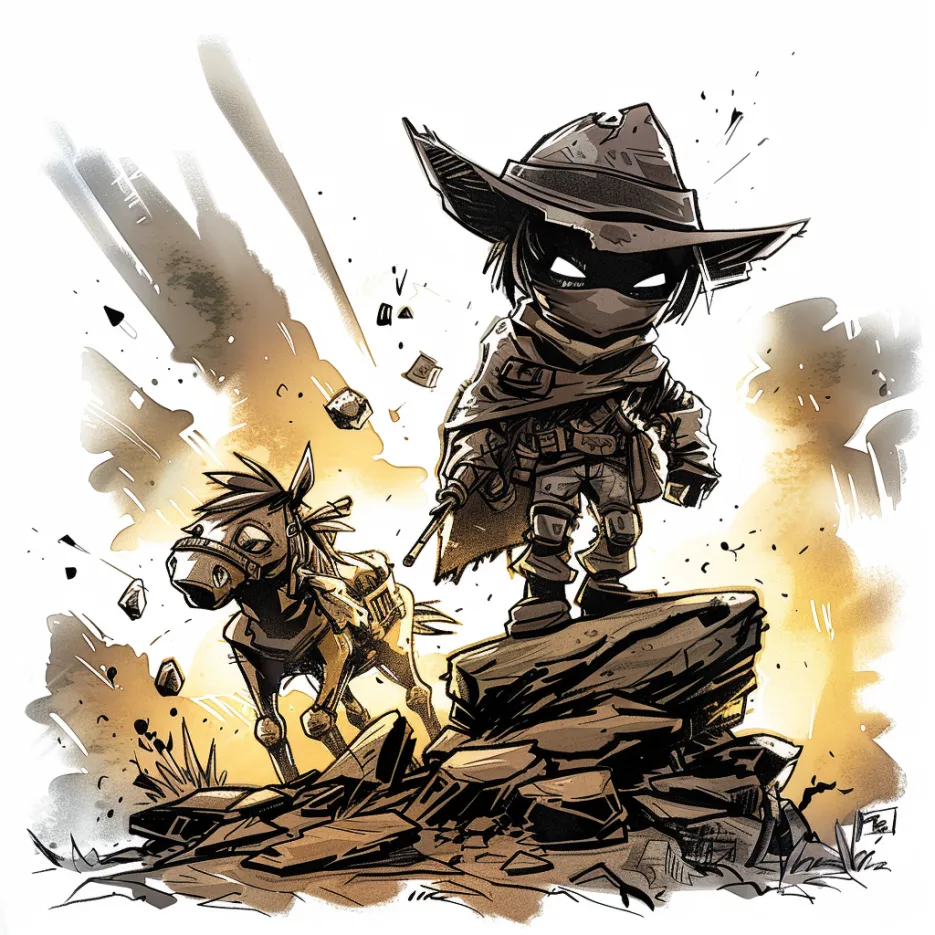